Move Position is a physics function that can be used to move a Rigidbody instantly.
It works in a similar way to moving an object using its transform component, except that it allows you change the position of an object that you might normally move with a physical force.
So how does it work? And when would you use it?
Usually, when you’re moving an object using physics, you might typically apply force to it to move it around in a realistic way.
But, what if you want to use the physics system but you want to move the object directly, in the same way as moving it using its transform component?
An example of this might be when you want to combine the functionality of the physics system, such as its gravity, with artificial movement.
Tight 2D controls, for example, can sometimes be easier to create by applying movement manually, since realistic physics can sometimes feel slow when compared to transform based movement.
But, if you want to use parts of the physics system, such as its gravity, or simulated collisions, simply moving the physics object using its transform isn’t the best way to do it.
This is because changing the position of a physics object doesn’t necessarily account for its movement, it simply teleports it to a new position and the physics system has to recalculate where it is in the next physics update. Something that, in older versions of Unity, was especially bad for performance.
While this does work, it means that the physics system never really knows where the object is going to be next and, as a result, rigidbody-specific features, such as interpolation, may work in an unusual way.
Instead, it’s possible to move a rigidbody object using the Move Position function, which is a rigidbody method that will move an object to a precise position in a similar way to setting its transform position.
Like this:
public class MovePositionExample : MonoBehaviour
{
public Rigidbody rb;
public float speed = 1;
Vector3 moveAxis;
void Update()
{
moveAxis = new Vector3(Input.GetAxis("Horizontal"), 0, Input.GetAxis("Vertical"));
moveAxis = Vector3.ClampMagnitude(moveAxis, 1);
}
private void FixedUpdate()
{
rb.MovePosition(rb.position + (moveAxis * speed * Time.fixedDeltaTime));
}
}
While this does work in a similar way to moving an object using its transform component, behind the scenes there’s a little more going on.
This is to do with the difference between moving a rigidbody object using its Rigidbody Position and the Move Position function.
Rigidbody Position vs Move Position
When you move an object in Unity, you’re not really moving it, you’re just frequently changing where it is.
For example, you can change the position of an object using its transform position which, when done over a number of frames, creates the illusion of movement.
Which works…
But, moving a rigidbody object in this way can be inefficient, since an object with a rigidbody attached to it is, technically, meant to moved using physics.
An alternative option is to change the rigidbody’s position instead.
Like this:
public class MovePositionExample : MonoBehaviour
{
public Rigidbody rb;
public float speed = 1;
Vector3 moveAxis;
void Update()
{
moveAxis = new Vector3(Input.GetAxis("Horizontal"), 0, Input.GetAxis("Vertical"));
moveAxis = Vector3.ClampMagnitude(moveAxis, 1);
}
private void FixedUpdate()
{
rb.position += moveAxis * speed * Time.fixedDeltaTime;
}
}
Basically, this does the same exact same this as changing the object’s transform position, except that it’s typically more efficient, since the physics system is aware of the move and doesn’t have to recalculate the position of its colliders.
However, the drawback is that the physics system usually runs at a slower frame rate than the game does, at 50 frames per second by default.
This means that physics-based movement can feel jerky, no matter how fast the game is actually running.
Normally, you would fix this by enabling interpolation, which smooths out a physics object’s movement in between fixed update calls.
For example, you could enable interpolation on important objects that need to move smoothly, such as the player, or a camera.
But, interpolation doesn’t work when moving a physics object by changing its rigidbody position.
This means that, if you want to create artificial movement, such as movement that’s not realistically simulated using Add Force, for example, your options are transform-based movement, which is smooth, but is inefficient and doesn’t work correctly with interpolation, or the rigidbody position method, which is accurate, but can’t be interpolated.
This is where the Move Position function is useful.
While it appears to work in the same way as changing its position, an object that is moved using Move Position is assumed to be moving from one position to the next.
The physics system knows that, in between fixed update calls, the object was moved, not teleported, from its last position to its current one, allowing it to interpolate the movement correctly.
Meaning that, so long as the object’s rigidbody is kinematic, and so long as you’ve enabled interpolation, the visual position of the object will be smoothed between the, slower, fixed update calls, even though the object is being moved manually and not with physics forces.
In an extreme example, with an artificially low fixed time step of 0.2 seconds, you can see the difference between the three methods:
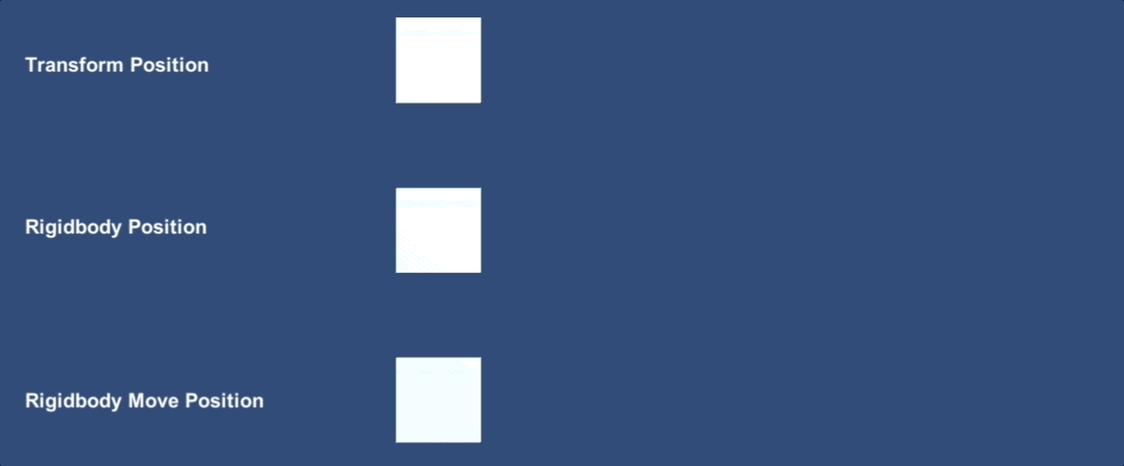
In this example, the transform method doesn’t work at all, while the rigidbody position method does work, but at the slower fixed update rate. Move Position, however, allows you to move an object manually while still using interpolation.
In this example, moving the physics object using its Transform Component doesn’t work properly. This is because the rigidbody’s interpolation is changing the position of the object, slowing it down incorrectly.
While moving the object using its Rigidbody Position does work, but at a much lower frame rate, since the object is only moved between fixed update calls and, although interpolation is enabled, it can’t be applied to the object because it’s technically being teleported, not moved.
However, when using a kinematic rigidbody, with interpolation enabled and using the Move Position function, the object is moved smoothly and precisely using physics, even though fixed update is only running at about 5 frames per second.
As a result, if you want to change the position of a physics object immediately, to teleport it somewhere else, set its Rigidbody Position value, but if you want to move it, gradually, and smoothly, but without applying simulated forces to it, use the Move Position function instead.