Rotating an object in Unity can be very straightforward.
However,
Just as there are many different ways to move an object, there are many different ways to rotate one too.
Which means that knowing the right method to use, in order to get the effect that you want, can be a little confusing at first.
But don’t worry, because in this in-depth guide I’ll show you all of the different methods for rotating an object in Unity, and the best time to use each of them, step by step.
Here’s what you’ll find on this page:
- How to rotate an object in Unity
- How to rotate an object around a point in Unity
- How to rotate a vector in Unity
- How to rotate the camera around an object in Unity
- How to rotate an object towards another
- How to rotate an object over time (Lerp & Slerp)
- How to Rotate a Rigidbody with the mouse
- How to create an FPS camera
- How to Rotate objects in 2D
Related Articles:
Rotation in Unity: Overview video
For a general overview of how to rotate in Unity, try my video, or continue to the full article below.
How to rotate an object in Unity
There are a lot of different ways to rotate an object in Unity.
Some methods are simple, some are more complex.
While others work best for certain tasks.
But don’t worry,
While there are a lot of different options available to you, many of them work in similar ways.
Which means that once you’re used to the basics of rotating an object in Unity, you’ll find it easier to use some of the more advanced rotation features that Unity offers.
So what is the basic method?
Rotation in Unity typically works by specifying an amount of rotation in degrees around the X, Y or Z axis of an object.
In the Inspector, you’ll see an object’s rotation as a Vector 3 value:
In the same way that a game object’s position in the world can be set using its Transform component…
Like this:
Vector3 newPosition = new Vector3(0, 10, 0);
transform.position = newPosition;
An object’s rotation can also be set directly via its Transform,
Like this:
Vector3 newRotation = new Vector3(0, 10, 0);
transform.eulerAngles = newRotation;
In this example, I’ve set the rotation of the object to 10 degrees around the Y Axis.
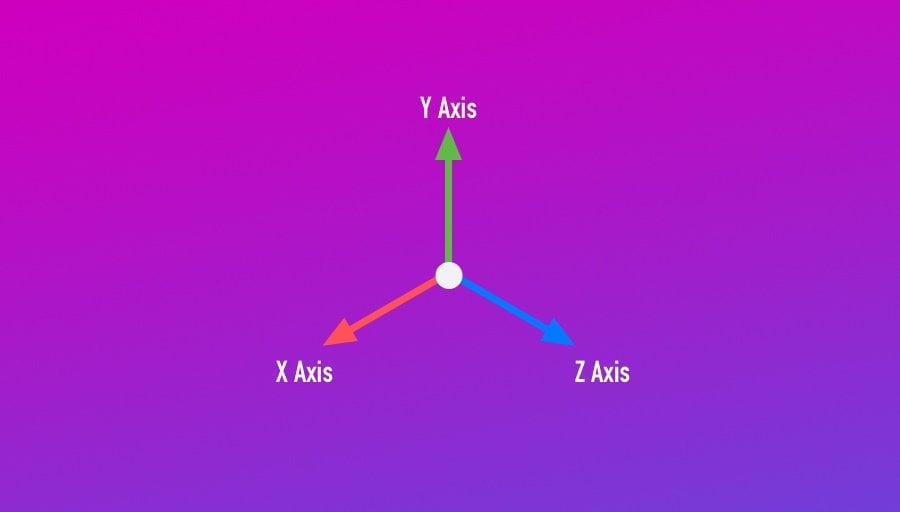
Rotational vector 3 values describe a degree of rotation around each of the 3 axes, X Y and Z.
Notice, however, that I didn’t set the rotation property directly like I might do when setting an object’s position, for example.
Instead, I set a different property, Euler Angles.
Why did I do it like that?
Behind the scenes, Unity calculates rotation using Quaternions, meaning that the rotation property of an object is not actually a Vector 3, it’s a Quaternion.
The rotation value you see in the Inspector is the real Quaternion rotation converted to a Vector 3 value, an Euler Angle, which is rotation expressed as an XYZ value for pitch, yaw and roll.
So, when using Transform.eulerAngles, you’re essentially setting and reading a converted value.
This means that if you want to set the rotation property directly, you’ll need to use a Quaternion value instead.
Like this:
// Copies another object's rotation
Quaternion objectRotation = otherObject.transform.rotation;
transform.rotation = objectRotation;
Or, instead of working in Quaternions, you can convert a Vector 3 Euler Angle rotation into a Quaternion.
Like this:
transform.rotation = Quaternion.Euler(new Vector3(0,10,0));
The Euler Angles property is a convenient way to set and read an object’s rotation in a format that’s easier to read and work with than Quaternions.
Quaternions vs Euler Angles in Unity
Should you use Quaternions or Euler Angles in Unity?
While Unity uses Quaternions behind the scenes, many of Unity’s rotation functions will allow you to use either type of value, either natively or by converting the value.
But which is better?
Generally speaking, Unity uses Quaternions because they’re efficient and avoid some of the problems of using Euler Angles, such as gimbal lock, which is the loss of a degree of movement when two axes are aligned the same way.
However, Quaternions are complex and aren’t designed to be worked with directly.
Euler Angles, on the other hand, are easier to read and work with.
Because of this, if you want to manually set a rotation, or provide a rotation amount in degrees, then it will be much easier to use an Euler Angle value to do so, and you’ll likely find that there’s an Euler based function available for you to do just that.
However, you may start to run into problems if you directly apply Euler Angle based rotation on a per axis basis or manually over time.
So what’s the answer?
It’s best to think of Euler Angles as a method of describing Quaternions in Unity.
They make reading and manually setting the rotation of an object easier to do.
However…
Trying to work exclusively in Euler Angles can cause unexpected behaviour.
For example, the converted Euler Angle value can vary, since the same Quaternion rotation can be described using different Euler values, or you might reintroduce gimbal lock by trying to work only with Euler Angles in local variables.
In practice, when using Unity’s built-in rotation functions, you shouldn’t need to worry about gimbal lock, even when passing in Euler Angles.
Unity will convert the Euler value and work with Quaternions behind the scenes, avoiding the typical problems associated with Eulers, while keeping their ease of use.
So while it’s generally better to use Quaternions when you can, there’s nothing wrong with using Euler Angles with Unity’s built-in rotation functions.
Setting the rotation of an object directly via its rotation property can be very straightforward.
However…
While this works well for setting an absolute rotation, one time, it’s not as useful for adding rotation to an object.
For example, to rotate an object by a set amount of degrees from its current orientation to a new one.
For that, you’ll need the Rotate function.
How to Rotate an object in Unity using the Rotate function
While setting the Euler Angles property of an object changes its absolute rotation, there will often be times when you want to add an amount of rotation to an object instead.
You might want to turn an object around by 90 degrees, or spin it on the spot, for example.
So how can you?
How to rotate an object by 90 degrees
It’s possible to add an amount of rotation to an object instead of setting its rotation value directly, in the same way that it’s possible to move an object by adding a vector to it, or by using the Translate function.
The easiest way to do this is by using the Rotate function.
Like this:
void RotateByDegrees()
{
Vector3 rotationToAdd = new Vector3(0, 90, 0);
transform.Rotate(rotationToAdd);
}
Instead of setting the rotation directly, like before, the Rotate function adds 90 degrees to the Y-Axis every time it’s called.
This can be useful for turning an object, changing its relative orientation or, if you apply an amount of rotation every frame, making it spin.
How to make an object spin
It’s possible to make an object spin on the spot by adding rotation to it every frame, scaling the rotation amount by delta time.
Like this:
float degreesPerSecond = 20;
private void Update()
{
transform.Rotate(new Vector3(0, degreesPerSecond, 0) * Time.deltaTime);
}
Multiplying the rotation amount by Time.deltaTime (which is the duration of the last frame) makes sure that the rotation is smooth and consistent, even if the framerate of the game changes.
Even if you’re a beginner, you may have already multiplied values by Delta Time before in Unity, as it’s usually required for any kind of function that occurs over time, such as movement, rotation or lerping. This is to make sure that, even if the framerate changes, the amount of movement over time remains the same.
In this case, it changes the amount of rotation from 20 degrees per frame to 20 degrees per second.
Which looks like this:
Rotate vs Rotation in Unity
Typically, Rotation in Unity refers to the rotation property of an object. In other words, the rotation values you see in the inspector, while Rotate is a function for adding an amount of rotation to an object.
So if you want to read the rotation of an object, or set it directly, you’d typically use the rotation property (or the Vector 3 shortcut: transform.eulerAngles). Whereas, if you want to apply an amount of rotation to an object, the Rotate function is designed to do exactly that.
Local rotation vs world rotation
An object’s rotation in Unity can be relative to the world or it can be local, where the rotation amount is relative to the rotation of its parent object.
Both an object’s local rotation and its absolute world rotation can be read as properties of the object’s transform.
Vector3 worldRotation = transform.eulerAngles;
Vector3 localRotation = transform.localEulerAngles;
A lot of the time, the local and world rotation of an object will be the same.
This is because, either the object that’s being rotated doesn’t have a parent object or because that parent object doesn’t have any rotation applied to it, in which case there’s no difference between the two values.
For example, if you rotate a child object by 90 degrees but don’t apply any rotation to the parent object, then the world rotation and the local rotation of the child object will both be the same.
This makes sense, because the child object has an amount of rotation applied to it which, when added to the parent’s rotation of zero, matches the object’s real rotation in the world.
However, if you only apply rotation to an object’s parent then the local rotation value for the child object will be different.
While the world rotation will match the parent, the local rotation will be zero, as no rotation is being applied by the child object’s transform.
Put simply, the world rotation value is the absolute rotation relative to world space. In this example, it’s the rotation of the parent object plus any locally applied rotation, if there is any. While the local rotation value is just the extra rotation added by that object’s Transform component.
So, which one should you use?
When working with rotation directly, for example when setting the rotation of an object via its Euler Angle property, you’ll have the option to set either its world rotation or its local rotation.
By default, when setting rotation directly, you’re setting the object’s world rotation:
// World Rotation
transform.eulerAngles = new Vector3(0,90,0);
Setting the world rotation of a child object will rotate it into that position absolutely.
This means that if the object has a parent with any rotation, the local rotation of the child will not be set to the new rotation value as you might expect. Instead, it will be set to a value that is equal to the difference between the parent’s rotation and the world rotation.
Basically, the local rotation will be set to whatever it needs to be in order to achieve the absolute world rotation you specified.
For example, if you set a child object to a world rotation of 50 degrees around an axis, and the object’s parent is already rotated by 10 degrees, the child object’s rotation will be set to 40 degrees, not the 50 you passed in.
The rotation of the object will technically be correct, you’ll get exactly what you asked for, but you may get some confusing results if you apply world rotation to child objects in this way.
So if you find that, when setting the rotation of an object via scripting, the Inspector values and the rotation don’t match, it might be because you’re setting the world rotation of a child object.
If, however, you only want to apply rotation locally, you can do that by using the local rotation value:
// Local Rotation
transform.localEulerAngles = new Vector3(0,90,0);
A lot of the time, this won’t make any difference.
When the object you rotate doesn’t have a parent, or if the parent isn’t rotated, using either method will produce the same effect.
However, there may be times when you’d like to position an object one way and rotate it another.
For example, if you want to spin an object that’s already rotated, like this titled chair:
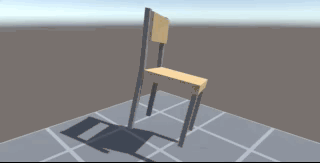
This chair is already rotated but spins on a flat Y-Axis.
One of the easiest ways to achieve this, and other rotation effects like it, is to simply nest the object inside another empty game object.
The child object can then be tilted to any local rotation you like, while the parent container can be automatically rotated on a flat Y-Axis in world space.
Separating the orientation of an object from its rotation like this can be useful for making rotation simpler to manage and easier to control.
Alternatively, if you don’t want to nest objects together, many of Unity’s built-in rotation functions, such as Transform Rotate can be overridden to operate in local or world space, recreating this effect, without the need for additional game objects.
How to rotate an object around a point in Unity
Rotating an object in Unity around one of its axes can be very simple.
For example, spinning a cube or turning an object upside-down can be very straightforward.
However…
As you start to rotate more complex objects, you may begin to notice that the position of the pivot point around which an object rotates is just as important as the rotation itself.
For example, if you build a basic door out of some primitive shapes, and then try to rotate it by 90 degrees to open it, you’ll quickly notice that something is wrong.
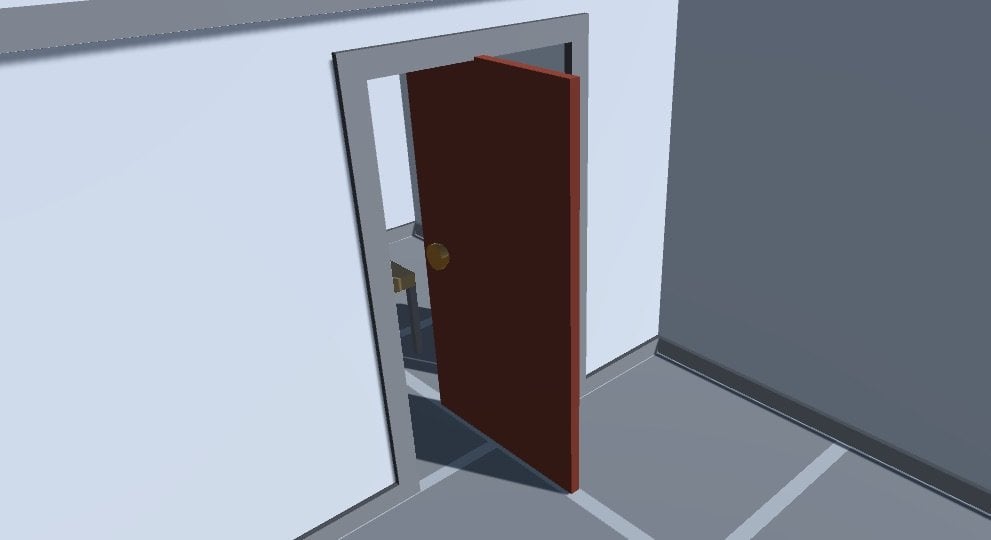
This is not how doors work.
In this example, I’ve built a door out of primitive objects, which means that the pivot point for the parent object, the main part of the door in my case, is in the centre.
This means that, when I rotate the object on its Y-Axis, it rotates around its centre.
Which, as I’m sure you’ve noticed, is not how most doors work.
So what can I do about it?
To fix it, I need to change the pivot point of the 3D object.
How to move the pivot point of a 3d object
Usually, when working with 3D objects that have been created using other tools, the pivot point of the object is set when the object is created.
For example, if I purchase a door model from the Asset Store, chances are that the pivot point will be in the correct place, on the door’s hinge.
I didn’t do that so, instead, I need to move the pivot point to a different position that allows me to rotate the door in the way I want.
Luckily it’s an easy problem to solve, by adding the game object you want to rotate as a child of another object that can act as a pivot point.
Here’s how:
- Place the object you want to rotate in position
- Next, create a new game object, the pivot, and place it at the pivot point of the rotation. Keep it separate while you’re positioning it.
- Finally, in the hierarchy, drag the object you want to rotate on to the pivot object, making it a child of the pivot
Then, instead of rotating the object directly, simply rotate the pivot.
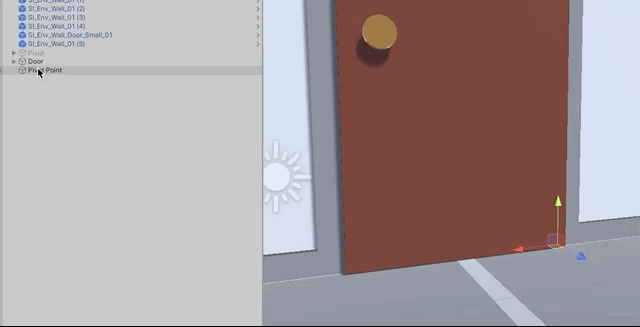
Creating a parent object is an easy way to change the pivot point in Unity
The same technique can be used to centre an object’s pivot point if, for whatever reason, it’s not already in the middle of your object.
You could even nest the root object (now the pivot) in another object that doesn’t rotate, and manage the local rotation of the pivot using a script.
Creating a parent object is an easy way to move the pivot point of an object.
However, it’s also possible to rotate an object around a point, without creating a parent object pivot, using Rotate Around.
How to use Rotate Around in Unity
Rotate Around works in a similar way to the parent object pivot method.
However, instead of creating a pivot point object and rotating it, the Rotate Around function takes a Vector 3 position, in world space, an axis to rotate around, and an angle in degrees.
Transform.RotateAround(Vector3 point, Vector3 axis, float angle);
This means that you can use Rotate Around to rotate an object either around a fixed point in world space,
Like this:
Vector3 pivotPoint = new Vector3 (-3.5f,0,0);
void RotateAroundPoint()
{
// Rotates around the pivot point and the Y-Axis by 90 degrees
transform.RotateAround(pivotPoint, Vector3.up, 90);
}
Or around another object entirely,
Like this:
public Transform objectToRotateAround;
void RotateAroundObject()
{
// Rotates around the pivot object's position and the Y-Axis by 90 degrees
transform.RotateAround(objectToRotateAround.position, Vector3.up, 90);
}
When using Rotate Around, the first argument, the Vector 3 point, is the pivot of the rotation while the 2nd Vector 3, the axis, decides which direction rotation will be applied.
This is important, as it determines which route around the point the object will rotate.
Finally, the float value, the angle, is the amount of rotation that will be applied in degrees.
How to define an axis of rotation in Unity
Several of Unity’s built-in rotation functions, such as Rotate Around, require you to enter an axis parameter in the form of a Vector 3 value, to determine the direction of the rotation that’s applied.
Entering a vector, using XYZ values, creates the definition of direction that’s used to determine the rotational axis.
For example, a vector of (1,0,0) would rotate around the X-Axis, while (0,1,0) rotates around the Y-Axis and (0,0,1) rotates around the Z-Axis.
Entering a mix of values creates a direction vector that’s proportionate to the values entered.
However, most of the time, you’ll probably only need to enter basic Axis values which, helpfully, can be accessed using Vector 3 shorthand, such as Vector3.left for the X-Axis, Vector3.up for the Y-Axis and Vector3.forward for the Z-Axis.
While these shortcuts represent basic directions relative to world space, it’s also possible to define an local directional value by referencing an object’s Transform component.
So, while Vector3.up represents the global up direction, Transform.up represents up in relation to a game object’s Transform.
Just like the Rotate function, Rotate Around is only applied once when it’s called, which means if you want to create continuous rotation, you’ll need to call Rotate Around in Update, multiplying the angle value by Delta Time.
Like this:
public Transform objectToOrbit;
void Update()
{
transform.RotateAround(objectToOrbit.position, Vector3.up, 10 * Time.deltaTime);
}
Rotate Around can be used to create rotation around other objects and points without using the parent object method, which can be useful for rotating an object around another, without needing to rotate both of them.
For example, I could use Rotate Around to create a basic orbit where the planet rotates around the sun and the planet’s moon rotates around the planet.
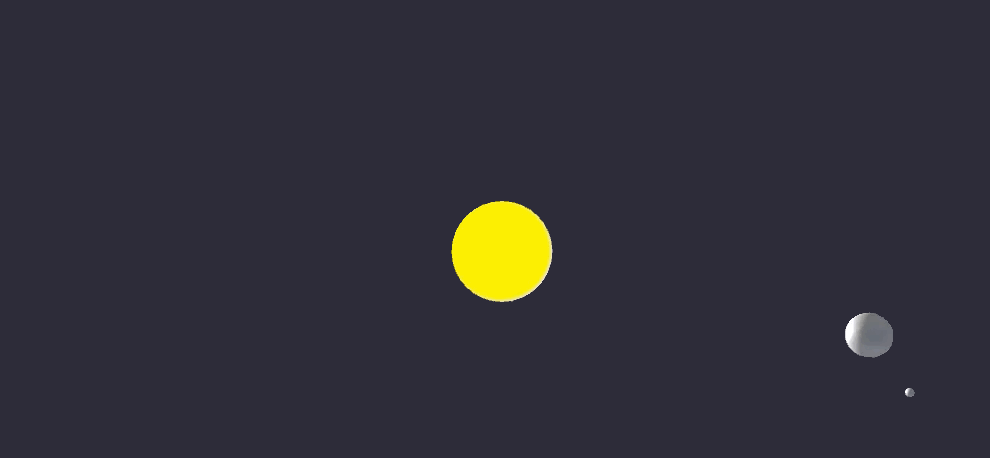
Rotate Around can be used to create a simple planetary orbit.
In this example, the moon is a child of the planet, which means that the moon’s position is being affected by the rotation of the planet.
While Rotate Around can be very useful and straightforward, there are a couple of drawbacks to using this method.
For example, if you use Rotate Around to move one object around another, and the object that’s being orbited moves, it’s going to affect the path of rotation.
And, while it’s possible to adjust the position of the object to avoid this, there’s another problem.
When using the Rotate Around function, the rotating object turns towards the point it’s rotating around.
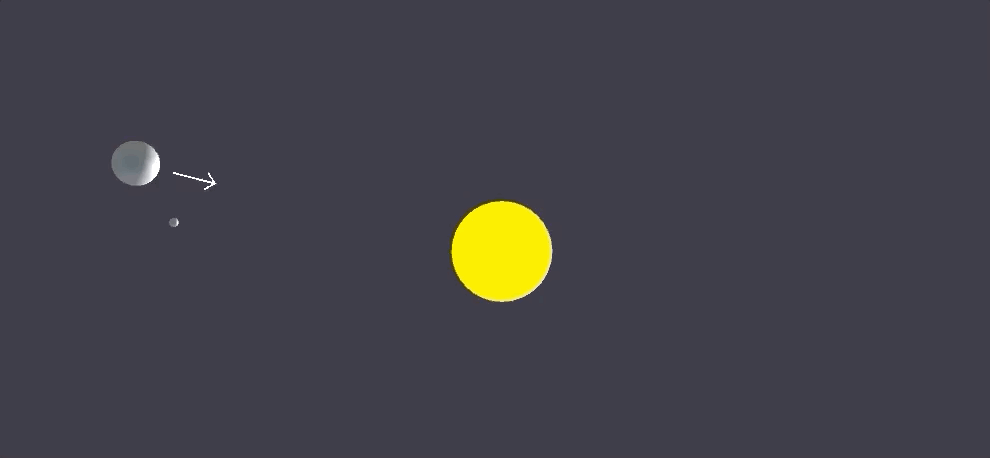
Using Rotate Around will also turn the orbiting object to face its point of rotation
This isn’t necessarily a bad thing.
In fact, it can be very useful, such as when rotating a camera around an object.
However, if you’re making an object orbit another, like in this example, it isn’t really how planetary orbits work.
Planets tend to spin around their own axis, while their position orbits around the sun.
So how can you rotate one object around another, without turning the object towards its point of orbit?
How can you rotate an object’s position around a point, without rotating the object itself?
Here’s how…
How to rotate a vector in Unity
A vector is a geometric value that defines a direction and length (which is the magnitude of the vector).
If you’ve worked with any kind of movement from one position to another, or done anything with Raycasts in Unity, then you will have used vectors before. Put simply, they describe direction and distance using Vector 3 values.
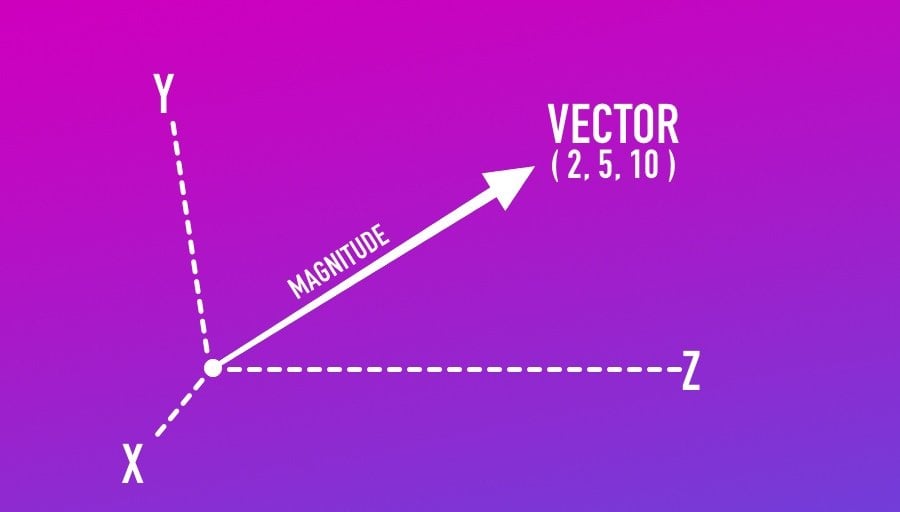
A vector is a definition of direction and distance (the vector’s magnitude).
However… did you know that you can also rotate a vector by multiplying it by a Quaternion?
This works by multiplying the Quaternion rotation by the vector you want to rotate.
Like this:
// Defines a point forward from the origin by 10 units.
Vector3 orbit = Vector3.forward * 10;
// Rotates the orbit vector by degrees around the Y-Axis
orbit = Quaternion.Euler(0, 10, 0) * orbit;
The resulting vector, which has now been rotated, can be used to calculate where the rotated object should be in relation to the object it’s orbiting.
Like this:
transform.position = objectToOrbit.transform.position + orbit;
Which means that, using vectors, it’s possible to rotate the position of one object around another in Unity, without actually rotating the object itself.
For example…
Let’s say that I want to orbit one object, a planet, around another, the sun, at a radius of 10 units and at a speed of 30 degrees per second.
First, I need a Vector.
In this case, all I want to do is define a direction and a radius, which will be the distance from the sun that the planet should orbit.
For that, I can multiply Vector3.forward, which is shorthand for (0,0,1) by 10, the radius.
That will give me a vector of (0,0,10).
I can then rotate the vector with an angle value, which I can add to every frame at 30 degrees per second, to create a circular orbit.
Like this:
public GameObject objectToOrbit;
public float angle;
public float radius = 10;
public float degreesPerSecond=30;
private void Update()
{
angle += degreesPerSecond * Time.deltaTime;
if (angle > 360)
{
angle -= 360;
}
Vector3 orbit = Vector3.forward * radius;
orbit = Quaternion.Euler(0, angle, 0) * orbit;
transform.position = objectToOrbit.transform.position + orbit;
}
What’s happening here is that I’m taking a basic forward vector and rotating it from its starting point every frame by the angle value.
In this case, I’m rotating around the Y-Axis, but I could also have entered different values to produce different results.
Note, however, that the order of multiplication matters. When Multiplying a vector by a Quaternion the Quaternion must go on the left, otherwise, this won’t work (it won’t even compile).
Finally, because the vector that I’ve created and rotated isn’t attached to anything (it basically describes a vector from the centre of the world), I’ve set it as an offset to the position of the orbited object, the Sun.
The result is a rotation of a vector between two virtual points, not objects, that calculates where one object should be in relation to another, creating a path of rotation. The orbiting object behaves as if it’s nested, however rotating the sun has no effect on the planet and it’s possible to rotate the planet independently of its orbit around the sun.
In this example, I only rotated around the global Y-Axis, which is useful for creating an easy orbit.
However, if I wanted to, I could also set the rotation to match a specific direction-based axis.
For example, imagine that the planet is 10 units away but is also several units higher than the sun and I wanted to create a rotation based on that existing direction.
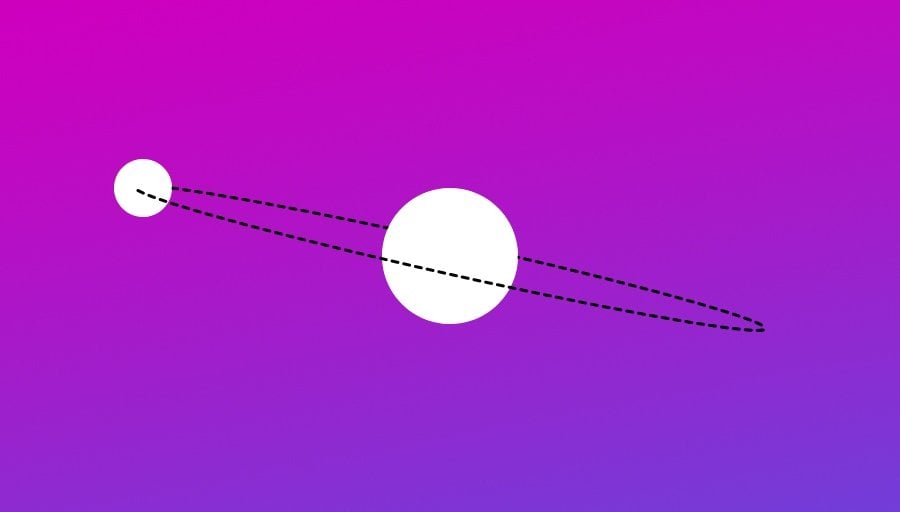
Getting the direction of the planet from the sun allows me to create an orbit on a tilt.
It’s possible to get a direction vector between two objects in Unity by subtracting their positions.
Like this:
Vector3 direction = transform.position - objectToOrbit.transform.position;
I can then calculate a plane of rotation based on the starting direction and distance of the object from its point of orbit.
Like this:
public class Orbit2 : MonoBehaviour
{
public GameObject objectToOrbit;
public Vector3 direction;
public float angle;
public float radius;
public float degreesPerSecond = 10;
private void Start()
{
direction = (transform.position - objectToOrbit.transform.position).normalized;
radius = Vector3.Distance(objectToOrbit.transform.position, transform.position);
}
private void Update()
{
angle += degreesPerSecond * Time.deltaTime;
if (angle > 360)
{
angle -= 360;
}
Vector3 orbit = Vector3.forward * radius;
orbit = Quaternion.LookRotation(direction) * Quaternion.Euler(0, angle, 0) * orbit;
transform.position = objectToOrbit.transform.position + orbit;
}
}
In this example, I’m measuring the direction and the distance of the object in Start, which gives me my orbit direction and radius.
I’m also normalising the vector, which limits its magnitude to 1. This basically limits the vector to directional information only, and while you don’t need to do this, I’m doing it so that I can multiply the direction vector by the radius variable, allowing me to change it later.
Once I have a direction vector, instead of rotating it around the world’s Y-Axis, which would cause the planet to orbit somewhere above the sun, I’m creating a new Quaternion angle using the Quaternion Look Rotation function instead. Look Rotation basically turns a direction vector into a Quaternion angle.
Then, to get the combined angle, I can multiply the Quaternion direction angle (which is the amount of tilt) by the angle of rotation (the angle float variable) to combine the two angles.
I’m still using the basic forward vector as a starting point, however now, instead of only multiplying it by the angle of rotation, I’m also rotating it by the angle of direction as well.
This is useful for creating automatic planet orbits as now, all I need to do is position the object relative to the point or object I want it to orbit, and the script will calculate a trajectory around that object.
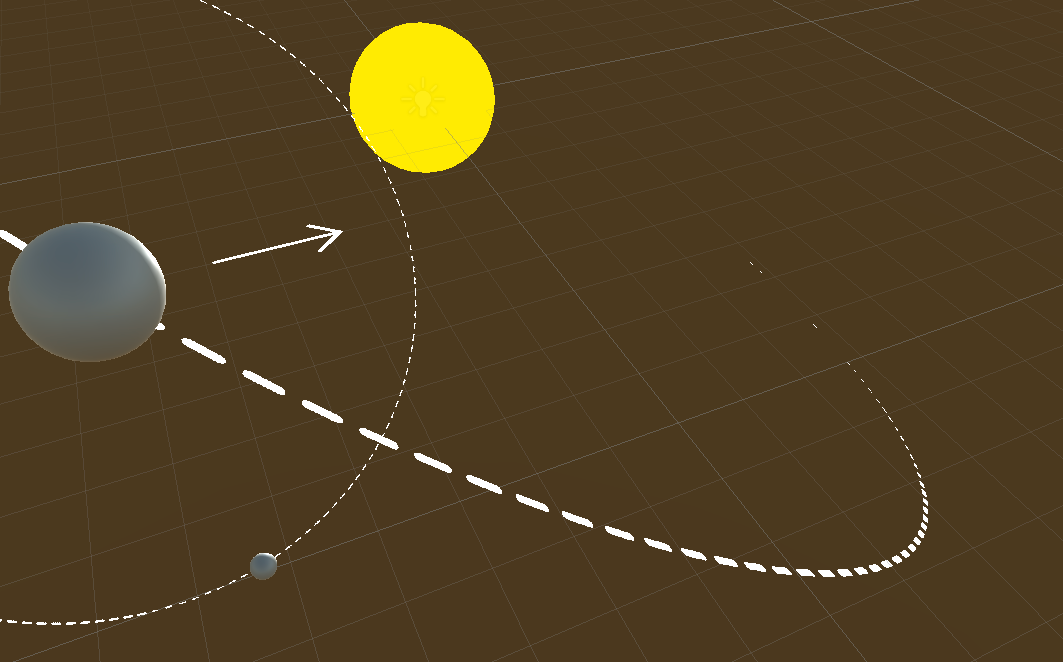
Planets can be given a more natural-looking orbit by rotating on an axis that’s based on their direction.
And if I want to change the axis of the orbit, I can do so by simply entering the angle into the X-Axis or the Z-axis instead.
This method is great for creating a path of rotation around a single axis.
However, there will often be times when you don’t want to just rotate one way around an object.
For example, what if you want to freely rotate a camera around the player using the mouse, or the keyboard, or using controller thumbsticks?
How can you pass input axes into a rotation function, to give the player control of the camera?
How to rotate the camera around an object in Unity
Being able to freely rotate the camera around an object with the mouse or other controls is a very common mechanic in many games; For example, to control the position of the camera around a player in 3rd-person.
In the previous example, moving a planet around the sun, I moved one object around another on a single plane of rotation, around the Y-Axis.
Rotating a camera around an object works in a similar way except, instead of a single fixed path, the player is able to freely move the camera around the object using two axes from the combined vertical and horizontal movements of the mouse, a controller thumbstick or keyboard keys.
So how do you do it?
One of the easiest ways to rotate the camera around an object is by using the Rotate Around function.
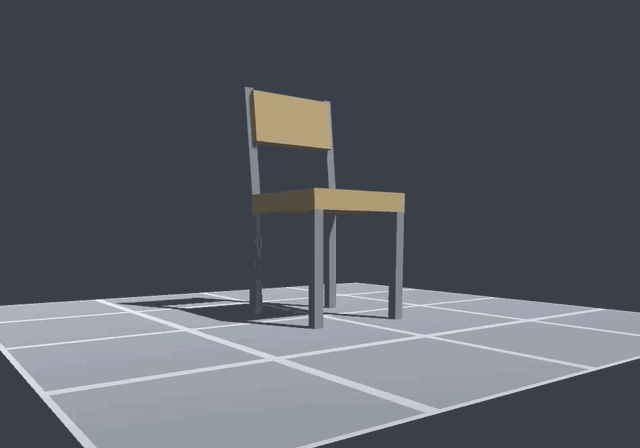
Using Rotate Around is an easy way to give the player camera control with the mouse.
The Rotate Around function rotates an object around another point, relative to its distance, while turning the object to face the pivot.
This makes it ideal for creating a mouse orbit camera, as it keeps the camera looking at the object.
Like this:
public class CameraRotateAround : MonoBehaviour
{
public Transform objectToOrbit;
public float radius;
void Update()
{
transform.position = objectToOrbit.position - (transform.forward * radius);
transform.RotateAround(objectToOrbit.position, Vector3.up, Input.GetAxis("Mouse X"));
transform.RotateAround(objectToOrbit.position, transform.right, Input.GetAxis("Mouse Y"));
}
}
This works, first, by moving the camera so that it faces the target object, but set back by a distance that’s equal to the radius variable.
Notice that I’m using the forward vector of the camera object that this script is attached to, not Vector3.forward, which is the world forward, to keep the camera in position.
Then, using the Rotate Around function, the horizontal input of the mouse (its X-Axis) rotates around the target object around the Y-Axis, which moves the camera left and right around the player.
While the vertical movement of the mouse moves the camera relative to its own X-Axis, moving it up and down.
Using Rotate Around, the object that’s being rotated will turn towards the point that it’s rotating around. For this particular use case, this is ideal, as it means that the camera will look towards the object it’s rotating around automatically, providing a simple method of rotating a camera around an object.
It works well and it’s straightforward.
However, it is also possible to rotate the camera around an object without using the Rotate Around function.
Here’s how to do that…
How to rotate the camera around an object without using Rotate Around
While the Rotate Around function is a straightforward way to rotate the camera around an object, it’s not the only method.
Just as it was possible to orbit an object around a single axis by rotating a direction vector, the same method can be used to create 360 spherical rotation around a player or object.
The benefit of using this method over Rotate Around is that the rotation is entirely position based. Neither the object or the pivot point will be rotated as a result, which can be useful if, for whatever reason, you want to move an object around another with the mouse, but don’t want the object itself to rotate.
Like before it works by defining a basic vector, such as the forward vector, and then rotating it into position using inputs from the mouse.
Like this:
public class Orbit3 : MonoBehaviour
{
public GameObject player;
public float angleX;
public float angleY;
public float radius = 10;
private void Update()
{
angleX += Input.GetAxis("Mouse X");
angleY = Mathf.Clamp(angleY -= Input.GetAxis("Mouse Y"), -89, 89);
radius = Mathf.Clamp(radius -= Input.mouseScrollDelta.y, 1, 10);
if (angleX > 360)
{
angleX -= 360;
}
else if (angleX < 0)
{
angleX += 360;
}
Vector3 orbit = Vector3.forward * radius;
orbit = Quaternion.Euler(angleY, angleX, 0) * orbit;
transform.position = player.transform.position + orbit;
transform.LookAt(player.transform.position);
}
}
In this example, the X and Y movements of the mouse are added to two angle variables, angle X and angle Y.
To limit vertical movement, the Y angle is clamped at 89 degrees in both directions, to stop the camera from going over the top of the player and round again.
The X angle is also checked to see if it has moved past 360 degrees, or has moved below 0 degrees, in order to keep the value within a normal range.
This check isn’t strictly necessary, as the rotation function will work just fine without it, however keeping the value within a typical rotation range prevents a potential, although highly unlikely, problem of reaching the limit of the value type, as well as making the value easy to understand at a glance.
Finally, a forward vector, multiplied by a distance that’s equal to the radius, is rotated by the two angles, and the position of the camera is then moved to match.
Because this method of moving a camera around an object doesn’t affect the object’s rotation, I’ve used the Transform Look At function, which turns an object to face the direction of a Transform.
In this case, the Look At function is helpful for making sure that the camera is actually facing the player way when it’s rotated.
However, it’s not the only option for turning one object towards another…
How to rotate an object towards another
There are several methods available for rotating one object towards another.
Which one you use depends on how you want the object to rotate towards its target.
How to use Look At
Transform Look At, which instantly snaps an object’s rotation to face the transform of another object, is probably the easiest method of rotating an object towards a specific point.
Like this:
public Transform objectToLookAt;
void Update()
{
transform.LookAt(objectToLookAt);
}
It takes a single Transform parameter, or a world position as a Vector 3, and will turn the object towards the target as soon as it’s called.
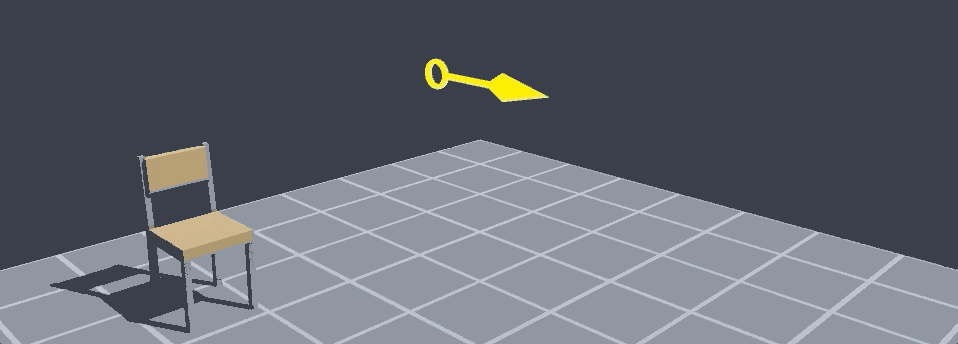
Look At is one of the easiest ways to make one object look at another.
Look At is great as a quick and easy way to snap an object’s rotation towards another object or towards another point in the world.
However, because it’s a function of an object’s Transform, it automatically rotates towards the object when you call it.
And while this can be extremely useful, there may be times when you want to calculate the rotation value required to look at an object, without actually looking at it.
The Quaternion Look Rotation function can help you do that.
How to use Look Rotation
Look Rotation basically turns a direction vector into a rotation value.
This can be useful for calculating the rotation value towards an object or point, but without actually turning the object to point at it.
For example, I could calculate the direction vector of two points by subtracting their positions.
Like this:
Vector3 direction = objectToLookAt.transform.position - transform.position;
I could then pass that direction vector into the Look Rotation function to get a matching Quaternion rotation value.
Like this:
Quaternion targetRotation = Quaternion.LookRotation(direction);
I now have the rotation required to look at an object as a Quaternion value.
Why would I want to do this?
Why wouldn’t I just use Look At to turn the object to its target?
While Look At can be used to instantly turn an object to face another object or point, depending on what you’re trying to do, you may not want the rotation to happen immediately.
Instead, you might want the object to rotate towards the direction of its target slowly and smoothly.
Following the direction of the target, instead of snapping to it.
And for that, Look Rotation can be used to give you a target value for an object to smoothly rotate towards.
So, now that you have a target, how can you smoothly rotate an object?
How to slowly rotate towards another object
Many examples of smoothly rotating an object towards another tend to use Lerp, which stands for linear interpolation, or Slerp, meaning spherical interpolation, to achieve this kind of eased movement.
However… this isn’t actually what interpolation functions such as Lerp and Slerp are used for.
Lerp and Slerp are typically used to carry out a single movement over a period of time, such as a rotation from one fixed position to another, or a movement between two points.
However, continuous movement, such as an arrow following the direction of a target, isn’t really what Lerp or Slerp are designed to do.
If using interpolation functions in this way gets you results that you like, then go ahead!
But… for continuously following the direction of a target, there’s a better function to use, Rotate Towards.
Here’s how…
How to use Rotate Towards
Rotate Towards is a Quaternion function that works in a similar way to Move Towards, which you may have used before to move an object closer to a target.
It looks like this:
Quaternion.RotateTowards(Quaternion startRotation, Quaternion targetRotation, float maxDegreesDelta);
In the same way that Move Towards moves an object towards a target position a little bit each frame, Rotate Towards turns an object towards a target rotation each frame, without overshooting when it gets there.
The result is a smoothed movement towards a target rotation.
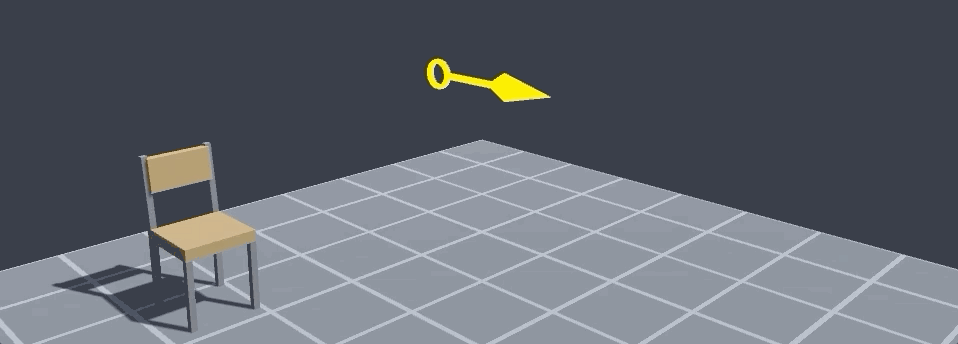
Rotate Towards turns an object towards a target each frame.
The target rotation is the direction vector of the object you want to rotate towards, which can be worked out by subtracting the target’s position from the object’s position.
The Look Rotation function then turns the direction vector into a Quaternion rotation.
Like this:
Vector3 direction = objectToLookAt.transform.position - transform.position;
Quaternion targetRotation = Quaternion.LookRotation(direction);
How fast Rotate Towards works depends on the Max Degrees Delta of the function, which limits how much the object can rotate in a single step.
Passing a degree value that’s multiplied by Delta Time into the Max Degrees Delta parameter sets the speed of the rotation in degrees per second.
Like this:
public Transform objectToLookAt;
void Update()
{
float degreesPerSecond = 90 * Time.deltaTime;
Vector3 direction = objectToLookAt.transform.position - transform.position;
Quaternion targetRotation = Quaternion.LookRotation(direction);
transform.rotation = Quaternion.RotateTowards(transform.rotation, targetRotation, degreesPerSecond);
}
The Rotate Toward function is ideal for continuous movement, as it’s always rotating towards a target at a specific speed.
However, if you want to perform a single rotation movement over a fixed amount of time, for example, to rotate an object 90 degrees over two seconds, then Lerp and Slerp can help you to do exactly that.
How to rotate an object over time (Lerp & Slerp)
While Rotate Towards continuously rotates an object towards a target at a set speed, you can use Lerp or Slerp to rotate an object by a fixed amount over a set period of time.
This works by taking a start rotation, such as the object’s own rotation at the start of the movement, a target rotation, which is where you want the object to end up, and a time value.
The time value, when calculated as time elapsed divided by duration, defines where the object should be during the movement. So, for example, at the halfway point (when time elapsed divided by duration equals 0.5) the rotation will be in the middle between the start and target rotations.
Then, once the movement has finished and the time has elapsed, the object is snapped into its final rotation.
So when might you use this?
Quaternion Lerp and Slerp are useful for rotating an object to a new rotation over a fixed amount of time.
For example, rotating an object around 90 degrees taking half a second,
Like this:
public class RotateObject : MonoBehaviour
{
float lerpDuration = 0.5f;
bool rotating;
void Update()
{
if (Input.GetMouseButtonDown(0) && !rotating)
{
StartCoroutine(Rotate90());
}
}
IEnumerator Rotate90()
{
rotating = true;
float timeElapsed = 0;
Quaternion startRotation = transform.rotation;
Quaternion targetRotation = transform.rotation * Quaternion.Euler(0, 90, 0);
while (timeElapsed < lerpDuration)
{
transform.rotation = Quaternion.Slerp(startRotation, targetRotation, timeElapsed / lerpDuration);
timeElapsed += Time.deltaTime;
yield return null;
}
transform.rotation = targetRotation;
rotating = false;
}
}
Because the rotation movement has a start, an end, and is carried out over a number of frames, it works best when placed in a Coroutine.
You may have also noticed that, in this example, I used Quaternion Slerp, not Lerp.
While the two functions are easily interchangeable, as both accept the same parameters, there is a slight difference between the two methods.
The difference between Lerp and Slerp for Rotation
When interpolating rotation, you’ll have the option to interpolate linearly, using Lerp, or spherically using Slerp.
Most of the time you’ll notice very little difference between the two methods. The main difference is that the length of the directional vector remains constant during Slerp while Lerp, which is linear, can be more efficient to perform.
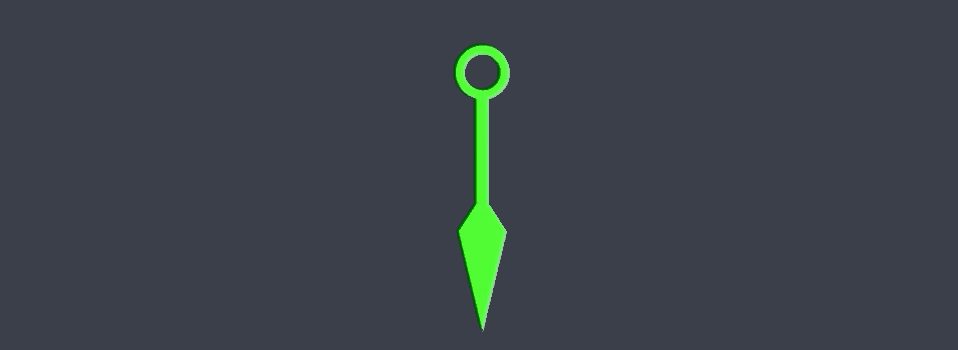
Lerp (green) vs Slerp (red)
So which should you use?
Generally speaking, using Slerp will produce a more accurate path of rotation, particularly when the difference between angles is large.
However… you may want to balance this against performance, as Lerp, which can be more efficient, will produce similar results in most cases and, for small angles, will barely be noticeable at all.
Quaternion Lerp and Slerp are best used for smoothly moving an object from one rotation to another.
However, like all of the previous examples in this guide, they work by setting the rotation of the object directly, basically emulating smooth movement with calculated values.
But what if you don’t want to directly set the orientation of the object?
What if, instead of rotating an object in a measured way, you actually want to spin it round with energy?
While rotation, just like movement, can be calculated and applied exactly, it’s also possible to apply rotation to a Rigidbody as a physical force.
Here’s how it works…
How to Rotate a Rigidbody with the mouse
Physics-based rotation, which is created using angular force and drag, can be a great way to create natural object rotation.
It works by applying an amount of force, which causes an object to rotate, after which angular drag slows the object down bringing it to a stop.
This can be used for all kinds of mechanics; Spinning an inventory object by clicking and dragging the mouse, for example.
So how can you use it?
How to use the Add Torque function
The Add Torque function adds an amount of rotational force to a Rigidbody, taking a Vector 3 value to define the direction of the rotation in world space:
Rigidbody.AddTorque(Vector3 torque);
So how can you use it?
Let’s say I want to create a floating object that can be spun around by clicking and dragging the mouse, similar to how you might view and rotate an item in a game.
For this to work, I’ll need to attach a Rigidbody to the object I want to spin.
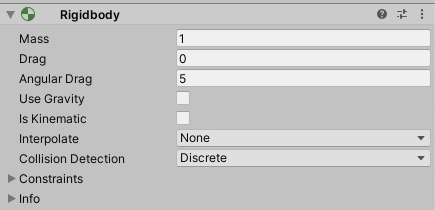
In this example, I’ve disabled gravity and increased the object’s angular drag.
I’ve turned off the Rigidbody’s gravity so that the object doesn’t fall out of the air, and I’ve given the object 5 units of angular drag so that it will stop fairly quickly after being rotated.
Then, to rotate the object using the mouse, all I need to do is pass in the Horizontal and Vertical axes of the mouse input into the Add Torque function whenever the mouse button is down.
Like this:
public class RotateRigidbody : MonoBehaviour
{
public Rigidbody rb;
public float strength = 100;
public float rotX;
public float rotY;
bool rotate;
private void Update()
{
if (Input.GetMouseButton(0))
{
rotate = true;
rotX = Input.GetAxis("Mouse X") * strength;
rotY = Input.GetAxis("Mouse Y") * strength;
}
else
{
rotate = false;
}
}
void FixedUpdate()
{
if (rotate)
{
rb.AddTorque(rotY, -rotX, 0);
}
}
}
When the mouse button is held down, I’m storing the directional movement values of the mouse in float variables and multiplying them by a strength value.
Then, in the Add Torque function, I’m applying the vertical mouse value to create rotational force around the X-Axis, while the horizontal mouse value creates force around the Y-Axis.
The end result is smooth, natural rotation, that’s applied when clicking and dragging the mouse.
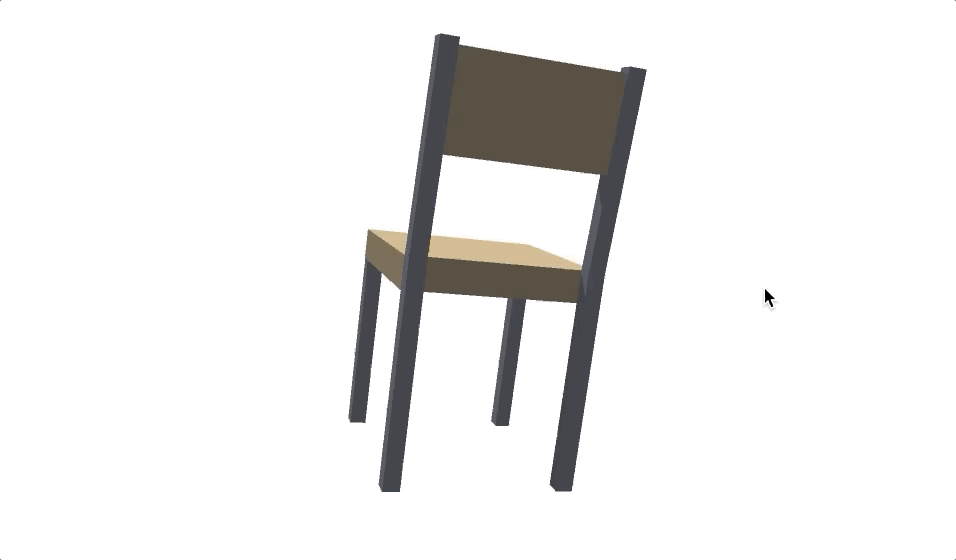
The Add Torque function is great for moving Physics objects around naturally with the mouse.
You’ll notice that I’ve split the functionality of this script across both the Update and Fixed Update calls, connecting the two indirectly using variables.
This is because, while the physics-based Add Torque function should be in Fixed Update, so that the application of force is in sync with the physics steps of the game, the input checks, which are framerate dependent, need to be in Update to work properly.
Splitting the functions like this prevents the different frequencies of Update and Fixed Update from affecting each other.
How to create an FPS camera in Unity
In the same way that it’s possible to use the mouse’s X and Y delta to apply force to a rigidbody object, you can also use the mouse’s input values to apply rotation to a camera object.
This allows you to tilt the camera up and down, around the X-Axis, or horizontally, around the Y-Axis, to turn.
It works by starting the camera at an X rotation of zero, meaning that it’s looking straight ahead, not up or down, and then simply adding or subtracting the input values of the mouse to a local Vector 3 value, the Look value.
The X value can then be clamped to a minimum and maximum degree of rotation, to prevent the player from looking too far up or down.
Finally, the Look value can be translated into a rotation using the Euler Angles property.
Like this:
public float lookSpeed = 1.5f;
Vector3 look;
void Update()
{
look += new Vector3(-Input.GetAxis("Mouse Y") * lookSpeed, Input.GetAxis("Mouse X") * lookSpeed, 0);
look.x = Mathf.Clamp(look.x, -80, 80);
transform.eulerAngles = look;
}
Keeping track of the rotation in a local Vector 3 is important, as it prevents you from having to check if the object is looking too far up or down using the converted Quaternion value.
This is because converting a Quaternion to an Euler can produce different results that, while not incorrect, could make it difficult to keep an object’s rotation within a set range.
Instead, keeping track of the input rotation before it’s even applied to the object makes it easy to control.
How to Rotate objects in 2D
A lot of the techniques used for rotating objects in 3D in Unity also apply when rotating in 2D.
This is because, generally speaking, normal game objects in Unity are the same in both 2D and 3D, with the only difference being that the forward vector, Z, usually represents depth in 2D.
Because of this, in order to get the results you want, it helps to be mindful of which axis an object is being rotated around.
Luckily, however, most rotation functions in Unity allow you to specify which way is considered to be up, allowing you to change the orientation of the rotation if you need to.
Such as the Look At function which, in this example is set to face the player in 2D, with the forward axis set as up.
public Transform player;
void Update()
{
transform.LookAt(player, Vector3.forward);
}
However, in some cases, when working with 2D objects, even changing the orientation of the rotation may not get you the results you want. In which case you may find it easier to simply rotate around the object’s Z-Axis using a single float value.
Which is, in fact, exactly how rotation works with 2D Rigidbody objects.
How to rotate a Rigidbody in 2D
While many objects rotate in similar ways in both 3D and 2D there are some specific differences when applying physics in 2D.
The 2D and 3D physics engines in Unity are separate. Which means that rotating a physics-based object in 2D can actually be much simpler than it is in 3D.
This is because true 2D rotation only occurs around a single axis. As a result, Rigidbody rotation is stored, and can be applied, using a single float value, which represents the amount of rotation in degrees clockwise or counter-clockwise.
Like this:
public Rigidbody2D rb;
void Update()
{
// spins a 2D physics object clockwise at 10 degrees per second
rb.AddTorque(10 * Time.deltaTime);
}
Comments
Hey John,
thanks for this great comprehensive guide. This went immediately to my bookmarks 🙂 and will be shared.
Cheers,
Jan
You’re welcome Jan, Thanks!
Hello John!
Very good article. I read it through, but still couldn’t find answer what I am looking for.
Maybe you could give a hint.
Let’s imagine a simple cube. User can rotate a cube by x, y, z axis.
When you rotate the object an example by Y axis, you cannot be so precise and also x, z axis are changing.
I would like to control the angel of movement per axis. If user intention was rotate by Y axis, I would like to understand users intentions (possibly seeing which of axis changed the most). Once I get that, I could make controlled movement by that axis by changing angle for certain degree and in certain speed.
How to catch the “user intention” when mouse is dragged to certain direction (or axis)?
My best guess would be that you might want to look at the user input and not the resulting rotation to work out what the user is trying to do. Obviously, it depends on how your mechanic is supposed to work, but I feel like the input would be easier to determine intent from. Hope that helps.
Awesome work, John. I’ve been a developer for almost 3 years now and I learn a new thing every time you post something. Loved re-learning rotation!
Thank you, great to hear it’s been helpful!
It was very rewarding to learn new things. Thank you!
You’re welcome Evan!
You simply fixed my broken understanding of Rotation in Unity. Thank you!
You’re welcome!
Hello John,
Great Resource!Keep it up.
My problem is I have 2 objects that rotate round a center point.The first object is a car that I control.I need a way for the second object(camera) to rotate around the center point at the same angle as the first object but on a wider orbit.For me RotateAround is not working as it keeps rotating.I want the camera to rotate only when the car moves(its like the camera is focused on the side part of the car).Any help would be greatly appreciated.
Thank and stay safe.
Thanks! You could try something like the rotating Vector method. where you can rotate around a point using an angle value. Something like this might work to get the angle of the car from an origin direction, such as forward. (Please note I haven’t tested this):
Vector3 carDirection = Vector3 carPosition - Vector3 center; float angle = Vector3.SignedAngle(Vector3.forward, Vector3 carDirection, Vector3.up);
Then you can use that angle to rotate a vector around the center at whatever distance you want. You’ll still need to make the camera look at the car though.
Hope that’s some help.
Please correct the code:
NOT:
transform.localEulerAngles = new Vector(0,90,0);
BUT:
transform.localEulerAngles = new Vector3(0,90,0);
Well spotted, thanks for that.
In the section “How to rotate a vector in Unity” can you explain why we need to use a basis vector (Vector3 orbit = Vector3.forward * radius) to multiply with the quaternions? What’s the difference if we instead use the displacement vector between the planet and the sun as the operand?
Anyway I’m really glad to have stumbled upon your blog. It’s like a treasure trove of Unity’s information.
Thank you! To answer your question, you could do that. In the first example in that section I use Vector3.forward for simplicity, however, I believe I used a direction vector in the example after that, where the orbit is tilted.
Very. Really. Helpful. Thanks, bro!
You’re welcome!
This helped me a lot. I am currently working on a game with a player doing twists and flips, so the fixed rotation of lerping and the rigidbody rotation just fitted perfectly for what I was looking for!
Thank you for also learning me about the stuff in such a good way!
That’s great! I’m really happy to hear how it helped you.
Great article! Bookmarked
Great article John thank you!
One question am I correct in thinking that if I only want to rotate around z in a 2d scene I could just adjust my transform like new Vector3(0f,0f,90f), I’m assuming we are safe from gimble lock in 2d like this?
Thanks,
LS
I wouldn’t have thought that rotating around one axis would cause any problems, however, you may still be better off using Quaternion Euler and passing in the rotation values that way.
Thank you
This is purely amazing. So much easier to understand what’s going on.
Thank you!
Hello John,
Thank you so much for taking your time to produce this article, it helped me a lot!
It’s the kind of content I couldn’t find in any course (expensive, btw).
Thanks! So glad it helped.
Hi John,
In my 2d project, I’m trying to turn the patrol’s direction in the direction it’s going,
I have read your article thoroughly and am watching with admiration.
but I still can’t find how to write a code
Hi Mehmet, what have you tried so far? Would you be willing to post your code?
Hey john
thank u so much for your time to make this article, it’s really helped me a lot. but i still have a problem, the thing is i want to make forklift pyhsics with lever and steering wheel. but i cant make the fork tilt a little when the lever is pulled down. it’s like when the lever pulled up the fork will tilt down to certain angle and when it’s already max it’s stop, and when the lever pulled down the fork will tilt up to certain angle and when already at min it’s stop.
thanks,
Eka
I think I understand what it is that you’re trying to make but I think that this is something that could be done a number of ways. For example, you might be able to do this with a physics joint, where the arm of the fork lift kind of wobbles in response to being moved but it depends on exactly what it is you want it to be like. Your best bet may be to make a quick sketch, or record a video of what you have so far, so that it’s super clear what you’re trying to do, and post it in the Unity forums. That way you’ll get a number of different solutions for what you’re trying to do. Hope that helps.
Hi, how can I use the function RotateTowards rotating only 1 axis, for example only the z axis? Thanks
You should be able to just pass in the angle of whichever axis you want when rotating using the Rotate function. e.g. transform.Rotate(new Vector3(0,90,0)); should twist an object by 90 degrees around the y-axis. Let me know if that’s not working for you.
public Transform objectToLookAt;
void Update()
{
float degreesPerSecond = 90 * Time.deltaTime;
Vector3 direction = objectToLookAt.transform.position – transform.position;
Quaternion targetRotation = Quaternion.LookRotation(direction);
transform.rotation = Quaternion.RotateTowards(transform.rotation, targetRotation, degreesPerSecond);
}
I meant with this code, how to rotate only in one axis towards the object.
Ah I see, in that case, so, if you didn’t want vertical rotation, for example, before you calculate the direction you could change the target object’s height to the same height as the origin. e.g. new Vector3(objectToLookAt.transform.position.x, transform.position.y, objectToLookAt.transform.position.z); Then it should only rotate on the x and z axes.
Thank you for your excellent explanations. So much in one place. This is my favourite reference now..
Thanks so much!
Great article! Very well explained, better than Unity documentation.
Thank you!
This and your lerping write up have been a tremendous help. Thank you so much!
You’re welcome Mike! Happy to help!
Awesome explanations! Thx!
You’re welcome Jorge!
Thanks a bunch, mate! After i learned your article any rotation for me is just a piece of cake. Appreciate your work!
Thank you! happy to help.
In my current project I had a task to instantiate and rotate kinematic rigidbody along path. And get transform properties to calculate positions for some object behind this object (using it’s transform.forward axis). So if I use “rb.MoveRotation(rotation)” to rotate instantiated object along path, then object appears in a scene with correct rotation, but in the next line of code I can’t get actual transform properties (it looks like object wasn’t rotated). I tried to set rotation usig line “transform.rotation = rotation”, in this case in the next lines of code I can get actual transform properties, but instantiated object with kinematic RB usually may have wrong rotation in a scene, it looks like sometimes RB ignores setting position and rotation using transform directly. I have found a function “Physics.SyncTransforms()” to sync transform with phycics engine. In this case I can set rotation using transform and set right rotation for the object after instantiantion from prefab in a scene and read transform properties in the next lines on code in a same function without using FixedUpdate(). And further I move and rotate instantiated object using rb.MovePosition() and rb.MoveRotation().
That’s interesting. As you mentioned in your comment, I’m assuming that this is to do with when physics updates actually occur.
Have you tried using Rigidbody.rotation to get the rotation after setting it instead of transform.rotation?
In my case I use parent transform and childs transforms (joints) for calculations after rotation (place behind and calculate offsets between it), I do not use angle of rotation.
Thank you so much for this! After a couple of years of guessing my way through anything related to rotations, this was exactly the basic-but-thorough explanation I needed to actually build my understanding!
You’re welcome Tim, glad it helped.
Awesome! Could you write about Playable in unity?It’s confusing for some beginer like me.Thank your wonderful article once again!
Thank you! Yes, I’ll look into playable.
Hey John! This has been really really helpful as a guide, thank you so much for this. So in my game I’m trying to get the player to rotate towards the ball when its in a certain radius. The problem is when it rotates, it completely messes up the movement input for the WASD keys because it’s now rotated to a brand new angle. How do I have my player turn and still keep my movement vectors in a clean up/down/left/right delineation?
You might want to try separating the movement and rotation to different objects. Have the root object as the one that moves, and then rotate a child object locally. Alternatively, don’t use local directions for movement, use global vectors or camera relative vectors, if that’s an option.
Hi John, thank you so much for your very helpful articles!
I’m a beginner. I ask you: In your code of the FPS Camera the user simply moves the mouse and everything works, the camera rotates; but how it’s possible to change that code so that only when the user is pressing the mouse button the camera rotates? And when the user releases the button the camera stops. Without conflicting with a normal mouse click or mouseButtonUp, for example to approach an object.
Many thanks!
Off the top of my head, if you wrap the code in an if condition for Get Mouse Button (not Get Mouse Button Down) that should work.
Hi John
Another great article!
I do have a use case though that I can’t seem to figure out yet. I have a Triangle shaped game object that I need the user to be able to rotate with the mouse. The method to rotate the game object is to place, what I call a “RotationRing” sprite, on the screen, that surrounds the game object to be rotated. Then when the user clicks and holds on the “RotationRing” and move the mouse, the “RotationRing” objects rotates based on rate of mouse movement and then rotates the game object that the “RotationRing” surrounds.
This works correctly when the “RotationRing” is placed on the game objects transform.position. However, for a triangle, this makes the “RotationRing” look off center of the game object. I have the Centroid position of the game object and I can position the “RotationRing” on the Centroid position. But using the same code to rotate the “RotationRing” and then the game object makes the game object move to a different position both while rotating and when the rotation is done.
I feel I am so close and probably just missing one element of how to get the game object to stay centered on its Centroid position while rotating but can’t seem to puzzle it out. So thought I would ask the Master :). If sending you the code lines I am using would help, just let me know.
Anxious to hear what you think.
Thanks
Steve
Thanks Steve, yeah if you could email me at [email protected] with your script, it would help me get a better idea and I’ll see what I can do to help.