Quitting the game in Unity can be a very simple task.
Which is great, because just about any game or application you make is probably going to need a way to exit at some point.
However, while the basic method of quitting a game in Unity is very simple, there are a few extra things to consider.
Such as quitting in the editor compared to quitting from a built version of the game, how to automatically run code when the game exits and how different platforms handle the closing of an application.
But don’t worry, because in this article you’ll learn everything you need to know about quitting a game in Unity, step by step.
So, how do you quit a game in Unity?
You can quit a game in Unity by calling the Application.Quit function, which will close a running application. However, while this works to end a built application, Application Quit is ignored when running the game in Play Mode in the editor.
In this article, I’ll show you how to properly use the Quit function, how to exit play mode from a script when working in the editor, and how you can run specific code when the application tries to close.
Let’s start with the basic method of quitting a game in Unity.
How to quit the game in Unity (using Application Quit)
You can quit a game in Unity using the Application.Quit function, which will close the running application.
Like this:
Application.Quit();
This works in built versions of the game, and can be used to give the player manual control over exiting the application.
Such as when a key is pressed for example.
How to quit when the Escape key is pressed
To trigger the quit function when a key is pressed, for example, the Escape key, simply check for the Key Down condition in Update and trigger the quit function when it’s pressed.
Like this:
public class QuitManager : MonoBehaviour
{
void Update()
{
if (Input.GetKeyDown(KeyCode.Escape))
{
Application.Quit();
}
}
}
Which will quit the game as soon as the Escape key is pressed.
However, while exiting when a key is pressed can be useful, chances are that you’ll be triggering the Quit function from a menu button instead.
So how can you connect the quit function to a button?
How to quit the game using a button
To trigger the quit function from a menu button, you’ll need to place it inside a public function.
Like this:
public void QuitGame()
{
Application.Quit();
}
Making the function public will allow the button to access the quit game method when it’s clicked.
Next, on the Button component that you want to use to trigger the quit function, add a new On Click Event:
And then drag the script containing the quit function to the empty On Click object field:
Then, to trigger the quit function when the button is pressed, select the quit script and then the quit function from the drop-down menu:
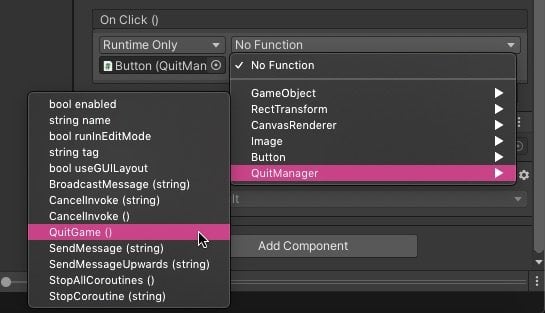
If you don’t see the quit game function in the list, make sure it’s a public method.
If you can’t find the function in this list, go back to the script to make sure that it’s definitely public.
Then, when you click the button, the game will close.
However… keep in mind, that this will only work from a built application.
In the Unity editor, the Application Quit function is ignored so pressing this button in Play Mode won’t do anything.
So, how can you add a quit function to your game that also works in the editor?
How to exit Play Mode from a script in Unity
While the Application Quit function works in a built game to close the application, it doesn’t do anything in the Unity editor.
The function is ignored, so trying to use the quit function to exit Play Mode, doesn’t work.
However, it is still possible to exit out of Play Mode from a script in Unity, you just need to use a different function to do it.
This works by setting the Is Playing property of the Editor Application class to false.
Like this:
UnityEditor.EditorApplication.isPlaying = false;
This has the same effect as quitting the game using Application Quit, except that it works in the editor.
This can be useful for testing the game quitting process without building the game first, as well as a shortcut for leaving Play Mode quickly.
For example, when a key is pressed.
Like this:
public class QuitManager : MonoBehaviour
{
void Update()
{
if (Input.GetKeyDown(KeyCode.Escape))
{
QuitGame();
}
}
public void QuitGame()
{
UnityEditor.EditorApplication.isPlaying = false;
}
}
Exiting Play Mode can be set up to work in the same way as the standard quit function, by placing it inside a method that’s called when a key or a button is pressed
There’s just one problem.
While setting Is Playing to false works to exit Play Mode in the editor, it won’t work in the built game.
In fact, because the Unity Editor class can’t be included in the built application, you won’t even be able to build the game when using this code.
And if you try, you’ll get an error.

Trying to include the Unity Editor class in a build will cause an error.
So how can you exit Play Mode from a script, without running into errors when you try to build your game?
How to run different code in the Unity Editor (using Preprocessor Directives)
Preprocessor Directives are used to conditionally compile code in your game.
In Unity, you can use preprocessor directives to run different code depending on certain conditions.
For example you could run different code depending on the platform the game is running on.
Or, if you’ve switched to the new Input System in Unity, you could ignore blocks of code that relate to the old Input Manager.
In this case, preprocessor directives are useful for checking if the game is being run in the editor or not.
This means that you can use a different quit method for when you’re working in the editor, and it will be automatically excluded when the time comes to build your game.
Like this:
public void QuitGame()
{
#if UNITY_EDITOR
UnityEditor.EditorApplication.isPlaying = false;
#endif
Application.Quit();
}
How to quit when Unity gets stuck in Play Mode
It can be easy to make a mistake in Unity, such as accidentally creating an infinite loop, which can cause the Unity editor to freeze in Play Mode.
This means that, if you haven’t saved the scene you’re working on, trying to exit Play Mode to recover your work may be impossible.
However,
While there isn’t currently a way to force-quit Play Mode in Unity, you may still be able to recover the work you lost if you have to force Unity to close.
Before entering Play Mode, Unity automatically saves a backup of the scene to the Temp folder. This means that, as long as you haven’t restarted the Unity editor, you can recover the scene you were working on before the crash, restoring your work.
Here’s how…
Inside the Temp folder, you’ll find a directory, “__Backupscenes”, which will contain a file called “0.backup”.
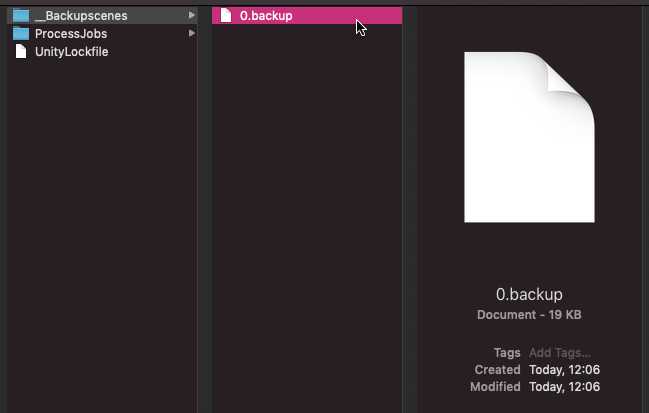
So long as you haven’t restarted Unity, you’ll find a backup of the scene you were working on in the Temp folder.
Changing the extension of this file to .unity will allow you to import it back into your project and open it as a scene.
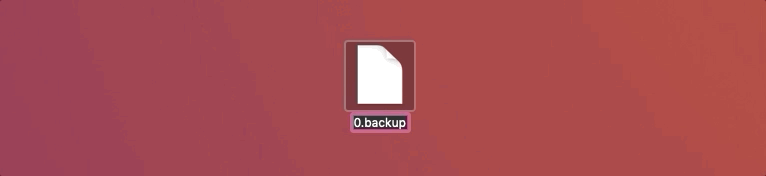
Change the backup’s extension to .unity to use it in your project.
Keep in mind however that the Temp folder will be cleared when Unity restarts.
This means that, if you open the editor before saving the backup file, or if you don’t move the backup out of the Temp folder, your work will be lost.
How to check if the application is quitting (using On Application Quit)
In the same way that you can run specific code at specific times, such as when an object is enabled or disabled, it’s also possible for a script to run specific code when the game quits.
This works in Unity by using the On Application Quit message, which is called on all game objects when the game is closed.
This can be useful for autosaving on exit, so that the player has the option of loading their game if they forgot to save it, or it could be used to keep track of how long it’s been since the application was last closed.
Like this:
void OnApplicationQuit()
{
PlayerPrefs.SetString("QuitTime", "The application last closed at: " + System.DateTime.Now);
}
In this example, a string that reads the current time is stored to a Player Pref value when the game quits.
If that same Player Pref is read when the application starts again, it’ll return the time that the application was closed.
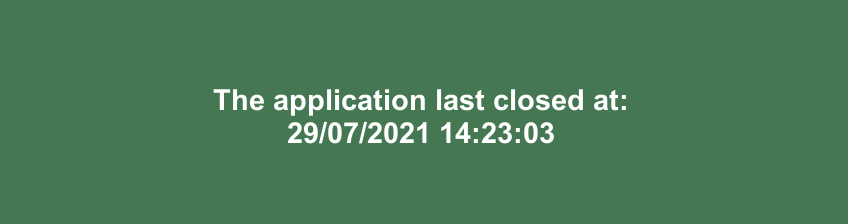
On Application Quit is called when the game closes, and can be used to run functions before quitting.
The On Application Quit message is called even if the script component that the function is on is disabled, but only if its game object is enabled.
While it will work when quitting manually from the game, or when quitting from the operating system, On Application Quit won’t be called if the application is forced to close or crashes.
Generally speaking, anything you add into the On Application Quit method should run before the game closes. However, if you try to start a Coroutine, you’ll find that it will be able to start but won’t complete.
For this reason, if you plan to use a coroutine as part of your quit process, for example, to fade out the screen, you may wish to place it before the Application.Quit function as part of the manual quitting process, so that you can run the coroutine in full before the game closes.
Keep in mind, however, that quitting the game outside of this process, such as by quitting from outside the application, won’t run the coroutine so, to avoid inconsistent results, make sure to place any important code, such as creating an exit save, inside the On Application Quit function.
How to prevent the game from quitting when Application Quit is called
While it’s possible to trigger a function when the game quits using the On Application Quit message, it’s also possible to prevent the game from quitting at all, using the Wants to Quit event.
Wants to Quit is an event that’s called when the quit process has been started, but can still be cancelled. It works by adding an event handler, that returns a true or false boolean value, to the Wants to Quit event. It’s then possible to prevent or allow the game to quit depending on the value that’s returned.
Like this:
public class PreventQuit : MonoBehaviour
{
public bool preventAppQuit;
void Start()
{
Application.wantsToQuit += WantsToQuit;
}
bool WantsToQuit()
{
return !preventAppQuit;
}
}
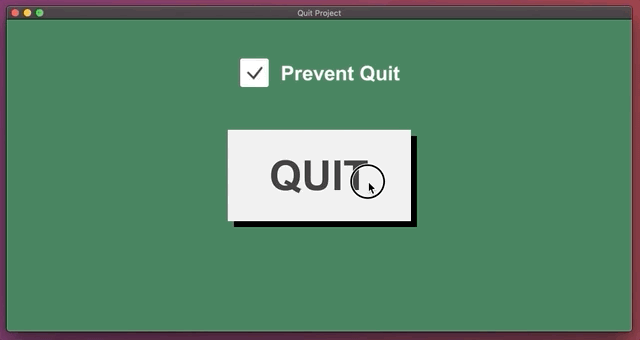
The Wants to Quit event can be used to prevent an application from closing.
Using Wants to Quit to prevent an application from closing can be useful for avoiding data loss.
However, while this method can be used to cancel a manual attempt to exit, it won’t prevent the game from quitting in the event of a forced close, a crash or on iOS, where closing an app can’t be prevented.
Quitting the game on mobile devices
Depending on the platform your game is running on, the process of quitting a game can be very different.
For example, on mobile devices, which manage their applications in a different way to desktop computers, you may need to change how the game is exited.
Such as on iOS, for example, where games are not actually meant to be closed at all.
How to quit the game on iOS in Unity
When running a game on iOS, you’re generally not expected to offer a method of closing the application.
This is because the method of closing an app on an iOS device is for the user to swipe up on its preview in the multi-tasking view, closing the application manually.
Whereas an iOS application that closes itself using the Application Quit function may, instead, appear to have crashed.
How to quit the game on Android in Unity
Using the Application Quit function on Android devices usually works as you expect it to.
However, when quitting an application you may still notice the game in the phone’s background apps list, as if it’s still running. This can sometimes be down to how the operating system manages recently used apps, keeping them in memory to make them easier to relaunch.
While your experience may vary, depending on the specific version of Android being used, it doesn’t necessarily mean that the game hasn’t closed as expected.
When offering methods for the player to trigger the Quit function on an Android device, you might want to assign the quit command to the phone’s Back Button.
This works by setting Back Button Leaves App to true…
Like this:
Input.backButtonLeavesApp = true;
Alternatively, if you want to make use of the Back button for quitting the game, but don’t want the application to immediately close as a result, you’ll need to manually implement the Back Button into your script.
On Android, the Back Button can be detected as the Escape key, so assigning a command to the Back Button can be done by simply checking to see if the Escape key has been pressed.
Like this:
if (Input.GetKeyDown(KeyCode.Escape))
{
// Back Button was pressed!
Quit();
}
Now it’s your turn
How are you quitting your game in Unity?
Are you running any code when the game closes?
And what tips do you have about exiting an application in Unity that you know others will find useful?
Whatever it is, let me know by leaving a comment.
Comments
Hi John. Great article. Learned a few new things from you.
Quick question: using the above examples, where would I actually add: Input.backButtonLeavesApp = true;
Thanks
Thanks! I’d suggest the best place to put it would be in an initialisation script in a starting scene at the beginning of the game.
That’s very helpful thanks John
You’re welcome!
Perfect