When you make a new empty script in Unity, you’ve probably noticed that it already contains some pre-written code.
Specifically, the Start and Update functions as well as three lines of code at the very top of your script file.
Which look like this:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ExampleScript : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
}
}
These are Using Directives, and what they do is allow a script to work with classes that exist in other Namespaces.
Even if you’re new to Unity, you’ve probably had to add using directives before, usually to work with a feature that requires a specific namespace, such as the UI system, or Audio Mixers.
Like this:
using UnityEngine.UI;
using UnityEngine.Audio;
But what are namespaces in Unity actually for, and should you be using them when you write scripts of your own?
What are Namespaces in Unity for?
The main purpose of a namespace is to avoid conflicts between classes that have the same name.
This is because duplicate classes, normally, cannot exist in the same Unity project, that is unless they exist in different namespaces, which allows you, and Unity, to identify which one is which.
For example, if you add a new script to an object called Player, and then try to add a second script with the same name, Unity will stop you, informing you that “a script called Player already exists at that path”.
This is because you’re basically trying to create a file with the same name in the same place, which is the default location for new scripts, the Assets folder.
But, even if you move the conflicting file to a different folder, Unity will still stop you from adding a duplicate script to an object, telling you that “a class called Player already exists”.
And if you create it in the project window, where Unity can’t stop you, you’ll get a compilation error telling you that “the namespace ‘<global namespace>’ already contains a definition for ‘Player'”.
So, on the surface, it seems like there’s no real way to create scripts with the same name in Unity.
That is, unless you use a namespace to do it.
How to create a Namespace in Unity
Namespaces can be created by wrapping one, or more, classes within a namespace container.
To do it, type the namespace keyword, followed by the name of the namespace and then add a body using brackets.
Then, simply place the class that you want to be included in the namespace inside the namespace body.
Like this:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
namespace GameDevBeginner
{
public class Player : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
}
}
}
This class can now be identified by its namespace, meaning that two classes of the same name can exist in the same project.
And when you need to add one of them to an object as a component, you’ll be able to see which is which.
Like this:
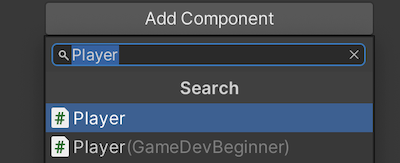
When two scripts have the same name, but they exist in separate namespaces, Unity will show you the namespace the script belongs to in parentheses.
However, even though two classes can exist in the same project, so long as they are contained within different namespaces, you still can’t keep two files with the same name in the same location.
Meaning that, if you’re going to create files with the same name, you’ll need to keep them in separate folders, no matter which namespace they’re in.
But why use namespaces at all?
Why not just give all of your scripts unique names in the first place?
When should you use a Namespace in Unity?
Generally speaking, the entire point of a namespace is to avoid a possible conflict with a script that has the same name.
Which, if you’re working on your own, might not seem like it’s something you need to worry about.
However, even if you’re not working with another person, it’s still possible for the names of your scripts to match new classes that are introduced by Unity in updates, or that are included in third-party assets purchased from the Asset Store.
Technically, this shouldn’t happen.
After all, the Asset Store Submission Guidelines require asset publishers to keep their named entities and identifiers in a separate namespace to avoid exactly this kind of problem.
However, it can still be a good idea to keep all of your code in a general namespace that’s specific to you anyway.
And, as you start to build the different systems in your game, you might find that it makes sense to use multiple namespaces or split a general namespace into subcategories.
Which can be useful for keeping your file names sensible, as it allows you to separate a subcategory of classes, such as Player Classes, without having to add the word player to every script you make.
How to create a Namespace hierarchy
It’s possible to create a namespace that exists inside of another namespace.
This works by typing out the hierarchical structure you want to create using the dot operator.
Like this:
namespace GDB.NamespaceOne
{
public class Player : MonoBehaviour
{
}
}
This allows you to further separate classes of the same name, but that still need to exist within their own namespace category.
When you add a class to a namespace, it won’t be accessible from other scripts like it would be normally.
Meaning that, if you just start typing the class name, you won’t get an auto-complete suggestion for it and, if you try to compile it you’ll get an error telling you that “the type or namespace could not be found”.
To fix this, you’ll need to identify which version of the class you want to use, by typing the name of the namespace before the class name and separated with the dot operator.
Like this:
public class Example : MonoBehaviour
{
GDB.Player player;
}
Or, if you’re going to be using the class reference repeatedly, it can be easier to add a Using Directive at the top of the script instead.
Unity using directives
Using directives in Unity allow you to specify that you’re using a particular namespace in a script.
Meaning that you won’t have to keep adding the namespace name ahead of a particular class name, you can just add the using directive once at the top of the script.
Like this:
using GDB;
public class Example : MonoBehaviour
{
Player player;
}
Keep in mind, however, that if you include two using directives that each include the same class name, you’ll get an Ambiguous Reference Error.
To fix this, you’ll need to specify which namespace each instance in your script actually refers to.
Like this:
using GDB;
using GDB.NamespaceOne;
public class Example : MonoBehaviour
{
GDB.Player playerOne;
GDB.NamespaceOne.Player playerTwo;
}
This can also happen when using a combination of Unity classes and built-in System classes.
For example, if you add the System using directive, which you typically need to do to use Actions, but you’re also using the Random class in your script to create random numbers, you’ll need to specify if you want to use System.Random or UnityEngine.Random.
Now it’s your turn
Now I want to hear from you.
How are you using namespaces in your project?
Are you using them everywhere?
Do you use sub-namespaces to organise your scripts?
Or do you find that you don’t need to use namespaces at all?
Whatever it is let me know by leaving a comment.
Comments
Excellent post. I use a namespace for my custom classes on the game I am developing. I do it because it’s a non conventional dungeon crawler and I manage it with my custom classes better, leaving Unity classes just to handle the interface.
Great piece. Concise and contains all one needs to know.
I don’t use namespaces because my projects are usually small but I think it will be fun to try it out in my latest project.
Thank you!
I like your post, but you might also have added ‘using alias directives’, to be a bit more complete, especially if you have a class of the same name in two different namespaces.
Thanks for the tip!
This also working when using C# <= 9?