Even if you’re very new to Unity, you’ve probably already used the Update function to call methods and run code that’s executed every frame.
You may also be familiar with Late Update, which works in exactly the same way as Update, except that it takes place after Update is called.
However…
While you may be well aware of what Late Update is and how it works, if you’re anything like me, you may not be 100% sure of when you’re supposed to use it and what could happen if you don’t.
But don’t worry, in this article I’ll show you how you can use Late Update, and other script execution techniques, to manage the order of events in your game.
So what exactly is Late Update, when should you use it and why is it so useful?
What is Late Update in Unity?
Late Update works just like Update in Unity.
Like Update, Late Update is called every frame on every enabled script, but with one key difference.
Late Update is always called after Update, meaning that Update will have been called on every script before the first Late Update is called.
This can be extremely useful, as it allows you to manage the order in which functions are run.
What is Late Update used for?
Late Update is used as a method of staging code execution, so that functions that depend on the outcome of other functions can be run after they’ve been completed.
This is important, because the order that code is executed can’t be guaranteed when using just Update alone, as the Update functions on different scripts and on different objects will all be called in an unknown order.
A lot of the time, this isn’t a problem.
However, if scripts interact with the same objects, or rely on each others’ data during the same frame, running them both at the same time in Update can cause unpredictable behaviour.
For example, in this scene I have a collection of blocks:
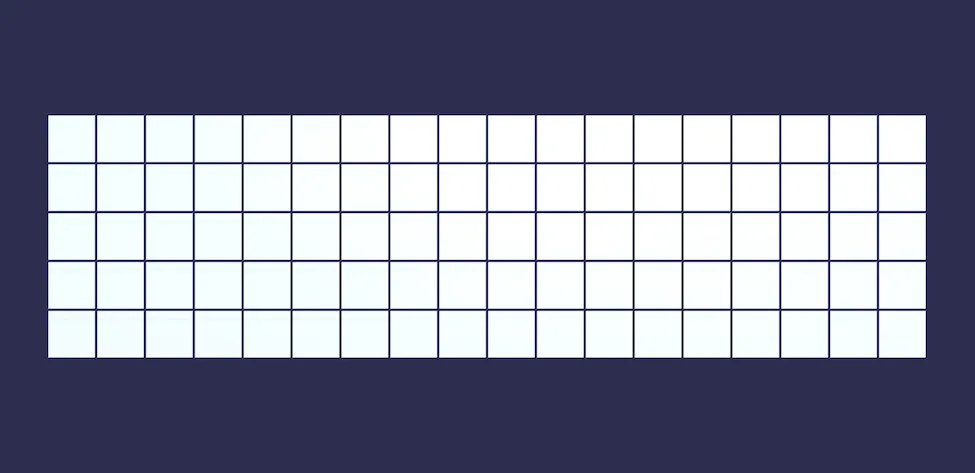
Each block has two scripts connected to it that are trying to change its colour.
Every block has two child objects, each with a script attached.
One of the scripts is trying to turn the block Red, while the other is trying to turn it Blue.
Like this:
public class TurnBlue : MonoBehaviour
{
public Renderer targetCube;
bool done;
void Update()
{
if (!done)
{
targetCube.material.color = Color.blue;
done = true;
}
}
}
Both scripts are trying to change the block in the Update function.
If the Red script runs after the Blue script, then the block will turn Red.
If the Blue script runs after the Red script, the block will turn Blue.
What actually happens, is that the blocks are turned to a mixture of Red and Blue, as each set of scripts have their Update functions executed in a different order.
Which looks like this:
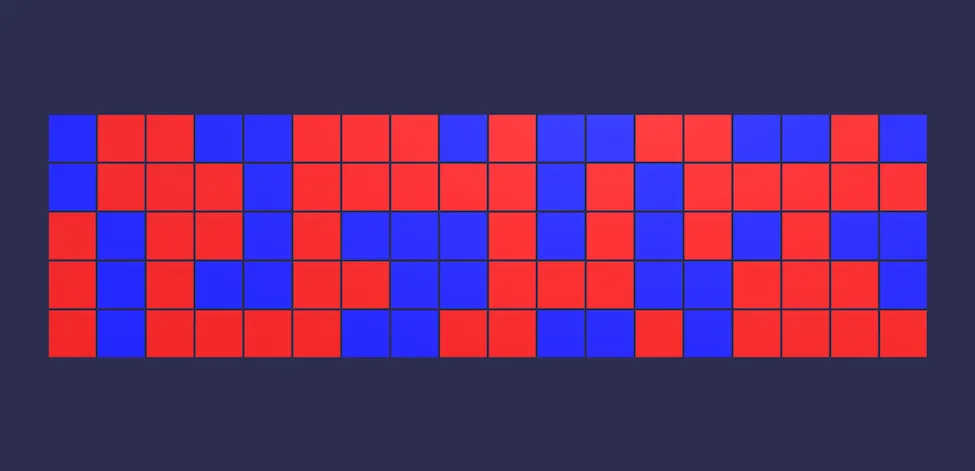
Each block has two competing scripts, both running in Update, trying to turn each block Blue or Red.
While it’s not necessarily random, it is unpredictable, as each script is trying to change the block at, essentially, the same time.
This creates a situation where the success of each script is determined by the order in which it is executed, leading to unreliable results.
However, this can be prevented by moving one of the scripts into the Late Update function.
Like this:
public class TurnBlue : MonoBehaviour
{
public Renderer targetCube;
bool done;
void LateUpdate()
{
if (!done)
{
targetCube.material.color = Color.blue;
done = true;
}
}
}
This means that all of the Red scripts will be run in Update first, and all of the Blue scripts will run in Late Update afterwards.
Meaning that every block will always turn Blue:
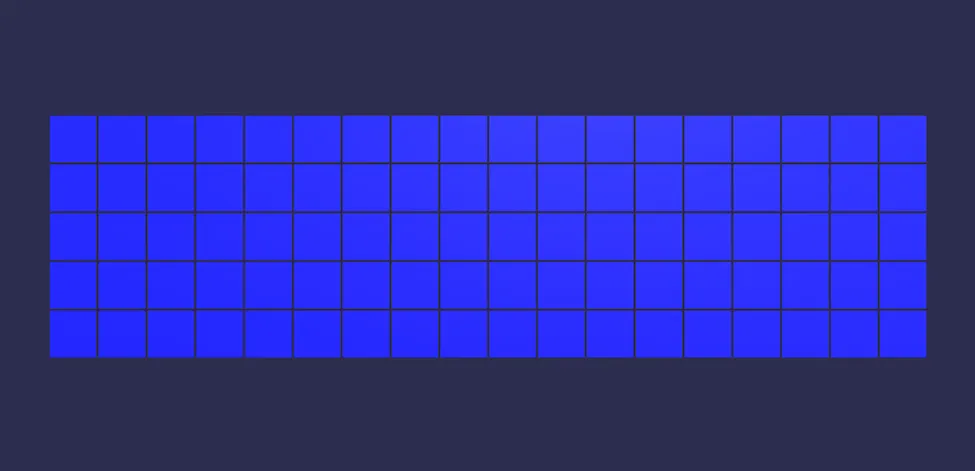
Moving the Blue script into Late Update guarantees that it is run after the Red script, turning every block Blue.
Using Late Update allows you to reliably run certain code after other functions.
But when would you actually use this?
And why is it important?
When would you use Late Update instead of Update?
The main reason for using Late Update is so that you can reliably act on the outcome of functions that were run in Update, during the same frame.
The example you’ll often see used is a camera system, where the position and rotation of the camera should be set in Late Update, so that the camera can always keep up with its target, the player, who may have moved during Update.
However, this isn’t the only reason you might use Late Update.
In fact, depending on how you’re structuring the logic in your game, using Late Update can help you to prevent bugs.
For example, imagine a game where the player can pick up and place objects on a grid.
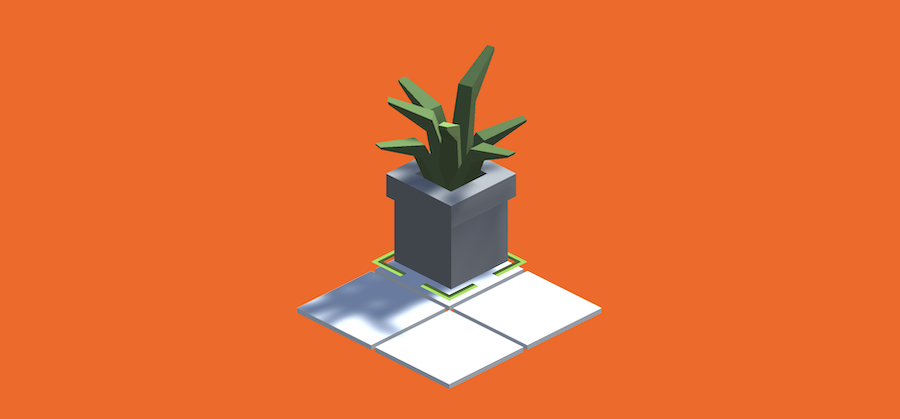
In this example, the player can use the cursor to pick up and place an object on any of the floor tiles.
A script on the object checks to see if the plant can be put down, while a script on the cursor waits for a mouse click and lets go of the object if it’s in a placeable position.
However, because both scripts are running in Update, there is a chance that the cursor’s drop function could happen before the plant checks its position.
In which case, if the player moves the cursor off of a tile, there will be a one frame window of time where the plant will wrongly report that it can be placed.
Which could cause the plant to be placed in an incorrect position.
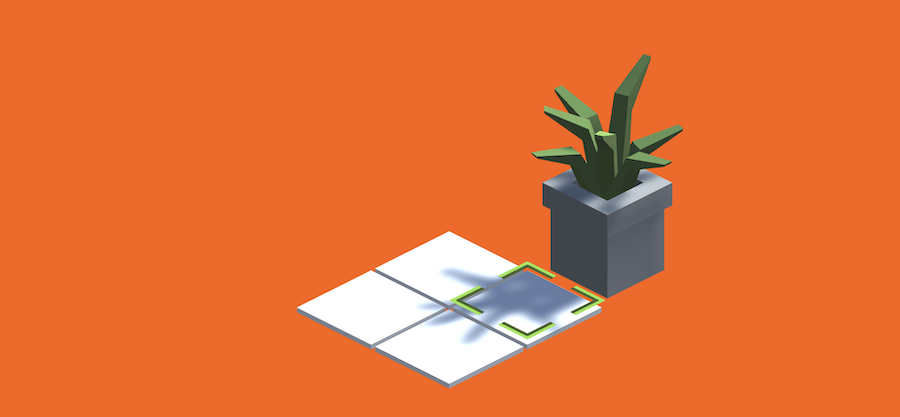
While it’s rare, trying to run codependent functions in Update can cause bugs.
Instead, placing the cursor’s drop function in Late Update would allow any other checks to take place first, so that the final check to see if the object can be placed is made with up to date information.
While it seems unlikely, bugs like this can happen when trying to run functions at the same time, and what’s worse, when they do happen, they’re often unpredictable and difficult to recreate.
Which makes them difficult to find and fix.
Which is why using Late Update to stage logical checks in your game can be so useful.
However, while Late Update can be useful for staging code to execute after Update, you may find that you need more control over the execution order of your scripts.
For example, what if you need functions to happen before Update?
What about physics? How can you manage the timing of Fixed Update calls, relative to other scripts?
And how can you manage the order of code in Coroutines?
Luckily, there’s a solution, because in Unity it’s possible to fine-tune the running order of specific scripts, relative to their normal time of execution.
Here’s how to do it…
Managing Script Execution Order in Unity
Unity executes event functions in a specific order which, generally speaking, looks like this:
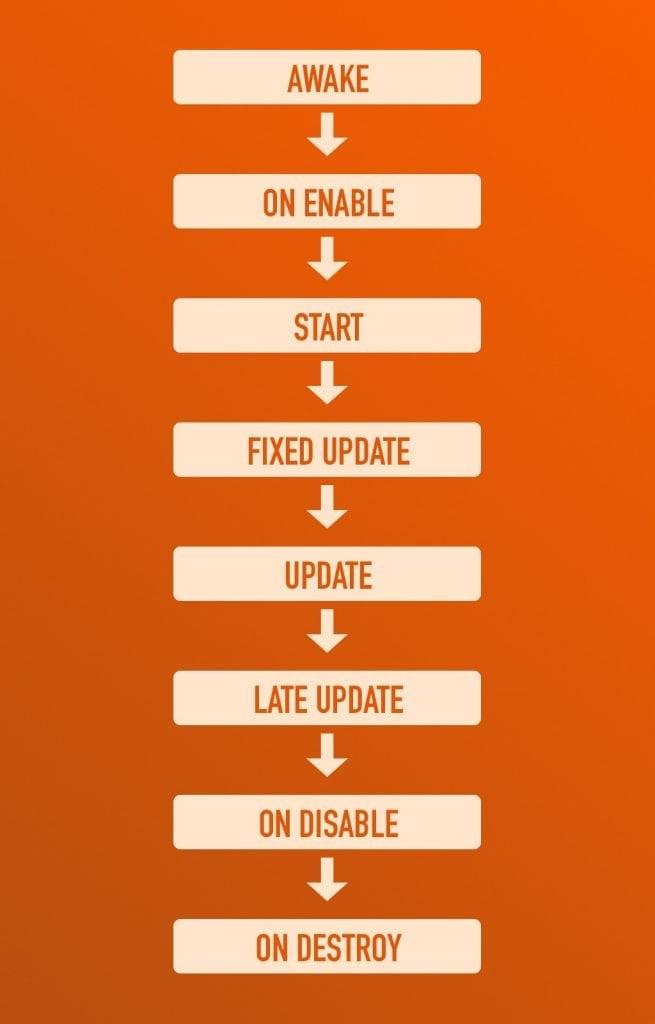
Unity calls event functions in a set order, which looks like this. (You can find a more detailed list in Unity’s documentation here).
Which is useful, because it allows you to understand the exact order of Unity’s event functions during a frame.
Moving logic to Late Update allows it to be run after Update because Late Update is a different event function that’s always called later than Update is.
But, apart from separating logic between the two events, how can you modify the order of scripts inside a specific event?
While the order of events can’t be changed, the order of specific scripts within each event can be set manually.
This works by modifying the Script Execution Order which can be found in Project Settings.
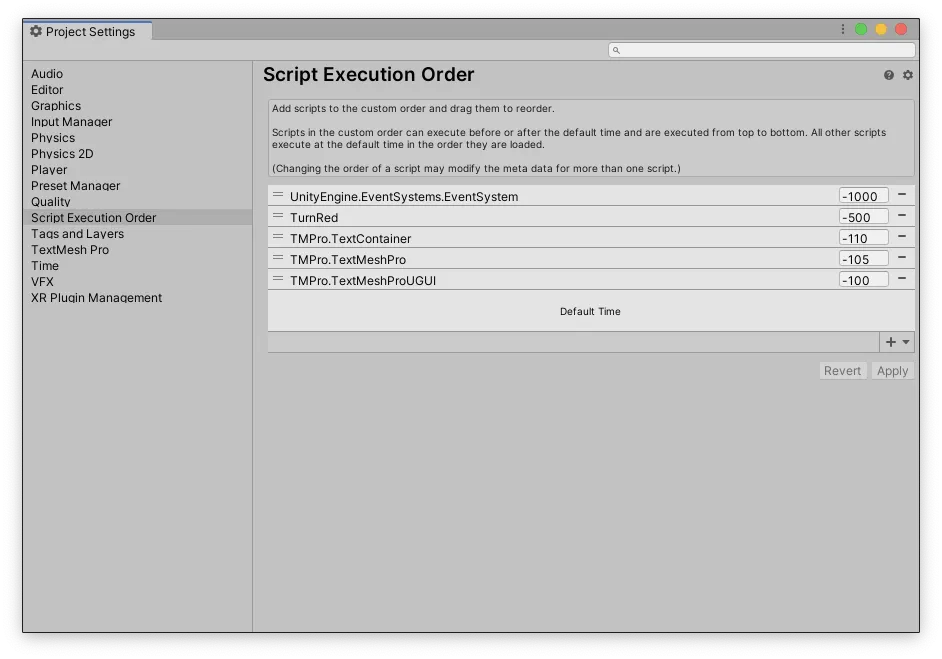
The Script Execution Order settings in Unity allows you to modify when a script is executed, relative to its Default Time.
The Script Execution Order processes scripts relative to their default time, which is the time they would normally have run, based on a relative value that is either positive or negative.
Negative values will cause a script to be processed early, while positive values will cause them to be processed late.
Which allows you to create any kind of execution order you want for a specific script inside of an event function.
For example, if you want a certain script’s code to be executed before any others, you could use the Script Execution Order to create an Early Update function.
Here’s how…
Early Update in Unity
While there isn’t a built-in Early Update function, it’s possible to prioritise certain scripts so that they run before others by changing their execution order.
This works by adding the script to the Script Execution Order list, and dragging it to the position you want.
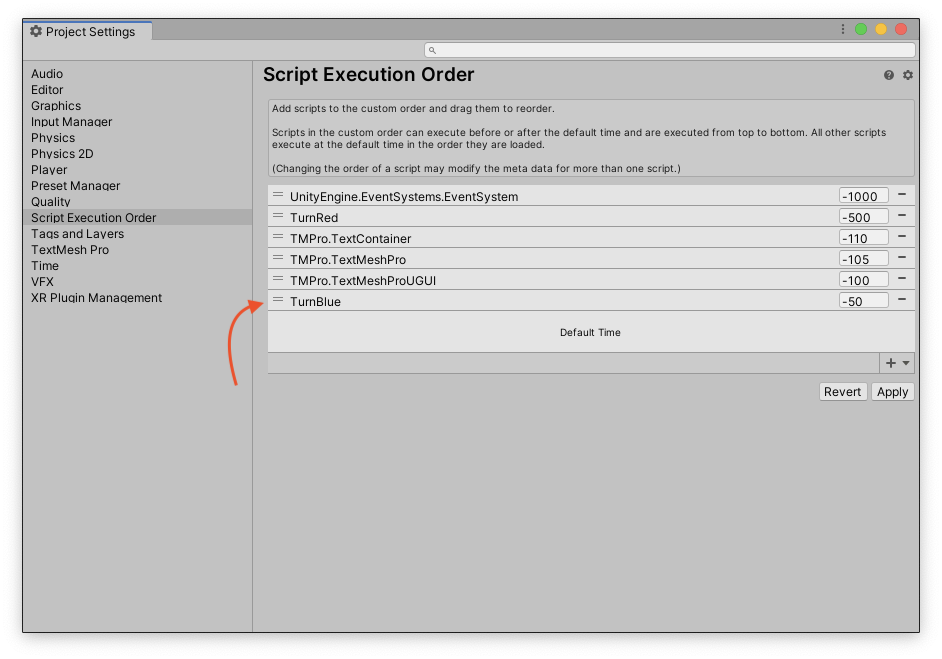
A negative execution order value will process a script earlier than others in the same event.
Exactly when the script runs will depend on the execution order value.
In this case, a negative value will execute the script before its Default Time, while a positive value will run the script after.
It’s important to note that the values themselves have no meaning, they only affect the position of a script in the list relative to other entries, allowing you to further order the early and late scripts.
Which is useful, as it allows you to control the execution order of important scripts, in fine detail, so that they happen in an order that works for your game.
The Default Time refers to the time that the script would normally have run, in this case, when Update would have been called for that script.
However, Update isn’t the only function that’s affected.
Adding a script to the Script Execution Order means that it will be called early or late for all of its events.
A script that’s first in Update, will also be first in Start, Late Update or even Fixed Update.
Which means that you can use the Script Execution Order method to create an early or late Fixed Update as well.
Late Fixed Update in Unity
Although there is a built-in Late Update event that is processed after Update, there isn’t any kind of built-in Late Fixed Update.
Which means that, if you want to stage the execution of physics-based scripts to run early or late, then you’ll need to use the Script Execution Order to do it.
Just like with Update, setting the script’s execution order value to a positive number will process the script’s Fixed Update function after all other Fixed Update functions, while setting it to a negative value will process it early.
Keep in mind, however, that changing a script’s Execution Order will not affect the order of the Fixed Update event, which is usually called earlier than Update and Late Update.
Instead, it simply means that a specific script’s Fixed Update function will be called before or after the Fixed Update functions on other scripts, but still all within the Fixed Update event.
Fixed Update is not Early Update
While Fixed Update is typically called before Update and Late Update it’s not the same as an Early Update, and shouldn’t really be used as one.
This is because Fixed Update, which is called in sync with the physics system’s fixed timestep, could be called more or less frequently than Update, which varies depending on the game’s framerate.
This means that Fixed Update is not a reliable event function for frame-based checks, as it may be called too often, or not at all, depending on how fast the game is running.
Managing the execution order of coroutines
Coroutines typically run alongside Update.
Which means that changing a script’s execution order will also affect the code inside a coroutine, running it early or late, relative to the Update functions of other scripts.
However…
This only applies while the coroutine is executing inside the Update event.
If a coroutine has yielded execution, meaning that it’s waiting, then the call to resume takes place in a different event function, that happens after Update.
What this means is that, any code after a yield statement will always be processed after Update, regardless of any changes to the Script Execution Order.
While the Script Execution Order does still apply, it will only affect the order of code being called in the same event.
Which in this case, will likely be the Yield Null event or Yield Wait For Seconds event, both of which take place after Update but before Late Update.
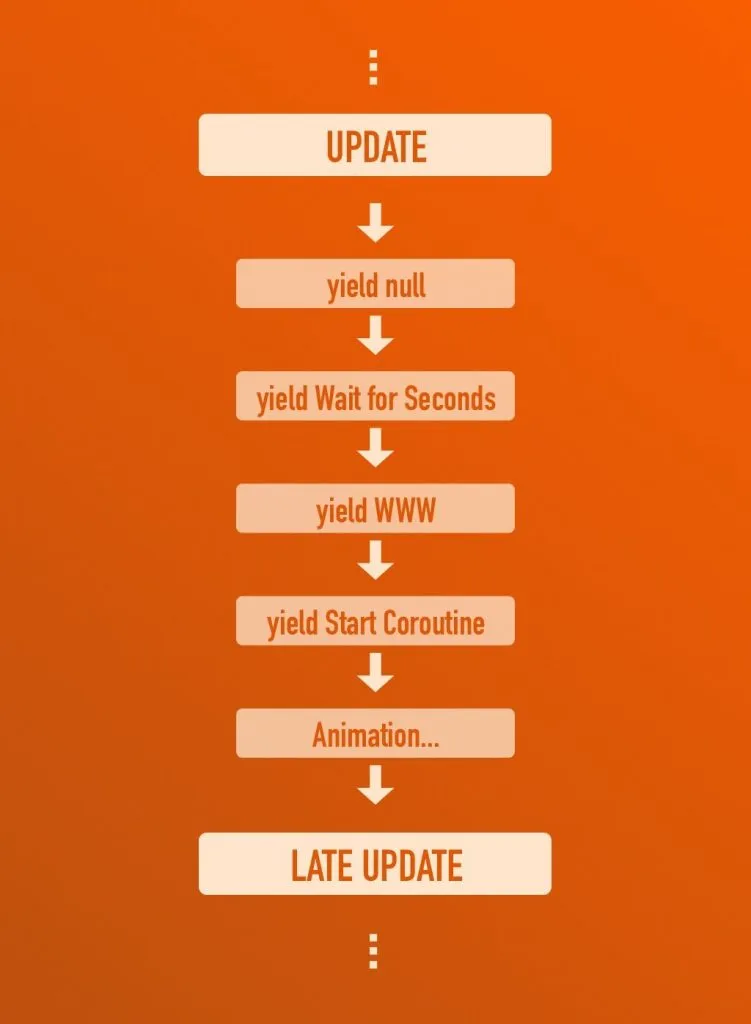
In between Update and Late Update, Unity calls a number of Coroutine Yield functions separately.
While this can make it tricky to order the execution of yielded code in relation to other events such as Update or Late Update, different Yield statements can be used to delay code from running until a certain point in the event order.
Such as Wait for Fixed Update, which is called after the next Fixed Update event.
Or Wait For End of Frame which, as one of the last events to be called, is useful for special code that requires an already rendered scene, such as taking a screenshot.
Now it’s your turn
Now I want to hear from you.
How are you using Late Update in your game?
Are you using the Script Execution Order to change the order of functions?
And what have you learned about Late Update that you know others will find useful?
Whatever it is, let me know by leaving a comment.
Comments
Great article John French, thanks a lot once again!
Here is my example; I had multiple blend trees for my character: idle, walk, run animations with different weapons or armless. I tried to find best transitions between blend trees for make them natural and realistic. When I push the switch weapon button, my character interestingly doing T pose in very first frame; and only rest frames playing as it should be.
Naturally I tried to fix animation transitions in Unity, animation transition in Blender, blend tree’s threshold values, my scripts, my monitor settings, my life style, my table’s decoration and nothing worked.
Couple of hours later, I was about to ask help. While I was explaining my problem, – I don’t know how- I remembered a tip from another forum and I switched my functions related to animations from Update to Late Update. And finally problem solved. I’m not exactly sure, but I guess methods related to animations should be defined in Late Update.
I wasn’t lucky enough to read your article before face with this problem. But I hope it helps to other fellas.
Thanks for sharing your experience.
Just a heads up, the header image for this page is broken.
Thanks, seems to be working fine, it might have been temporary or local to you.
Thanks for this article. Very helpful. Subscribed.
Thank you!
This article was incredibly helpful. Thank you for making this blog, it’s the best of its kind out there, by far.
Thanks so much!