There are many different ways to control a game.
By using a controller, by touching a screen or, as is the case with many PC games, by using the mouse in some way.
However, not every game uses the mouse and even the ones that do may modify how the mouse cursor appears, either by replacing it with a custom cursor, hiding it out of view, or by locking it to the centre of the screen, such as for a first-person game, where the mouse is typically used to control the orientation of the player’s head.
Luckily, however, locking or hiding the cursor in Unity is pretty simple…
So how does it work?
Hiding the cursor in Unity works by setting the Visible property of the Cursor Class to false, while setting the cursor’s Lock State to locked will force the cursor to the centre of the screen while the application is in focus.
Which is useful, as it means locking the cursor for a first-person game, or hiding the cursor because you want to use your own custom pointer, is easy to do.
However, while this method is simple, there are a couple of common mistakes to watch out for when writing a script that will modify the behaviour of the mouse.
In this article, you’ll learn how the basic method works and how to fix the few minor issues that might pop up when working with the cursor in Unity.
Here’s what you’ll find on this page:
Let’s get started…
How to hide the cursor in Unity
Hiding the cursor in Unity is, helpfully, very simple to do.
Just set the Visible property of the cursor class to false to hide the cursor, or to true to show it again.
Like this:
void Start()
{
// Hides the cursor...
Cursor.visible = false;
}
This will hide the operating system’s hardware cursor when the application is in focus.
Which can be useful if you want to replace the system cursor with an in-game object that follows the mouse around.
Hiding & revealing the mouse in the editor
When working in the editor, the Game View will start in focus when play mode is activated, meaning that, as soon as you start the game, the cursor will be hidden.
Which, if you haven’t hidden the cursor in Unity before, can make finding your mouse again, such as to leave play mode for example, a little tricky to do.
While it’s possible to click on a different part of the editor, outside of the game view, to shift focus away from it, this might be difficult if you can’t see where it is.
To find the cursor more easily, pressing Escape while in play mode will shift focus out of the game view, back to the editor, revealing the mouse’s position.
Why is Cursor.visible not working
If you’re setting Cursor.visible to true, but the cursor isn’t appearing, then it may be because you’re trying to lock the cursor and force it to display it at the same time.
While this may not be the behaviour that you want, this is the normal behaviour of the cursor class when it’s in a locked state.
Locking the cursor will automatically hide it, meaning that it will be invisible, no matter what cursor visible is set to.
How to lock the cursor in Unity
In Unity, it’s possible to limit the on-screen movement of the mouse using the Cursor Lock State, which can be used to limit the cursor to the bounds of the game window or to completely lock it in place at the centre of your game’s screen.
This works by setting the Lock State to one of the 3 predefined Cursor Lock Modes:
- None, which is the default, unlocked behaviour of the cursor.
- Locked, which will lock the cursor to the centre of the screen.
- Confined, which limits the movement of the cursor to inside the game window only
Like this:
void Start()
{
// Releases the cursor
Cursor.lockState = CursorLockMode.None;
// Locks the cursor
Cursor.lockState = CursorLockMode.Locked;
// Confines the cursor
Cursor.lockState = CursorLockMode.Confined;
}
Locking the cursor will force it to the centre of the screen whenever the application, or the game view when you’re working in the editor, is in focus.
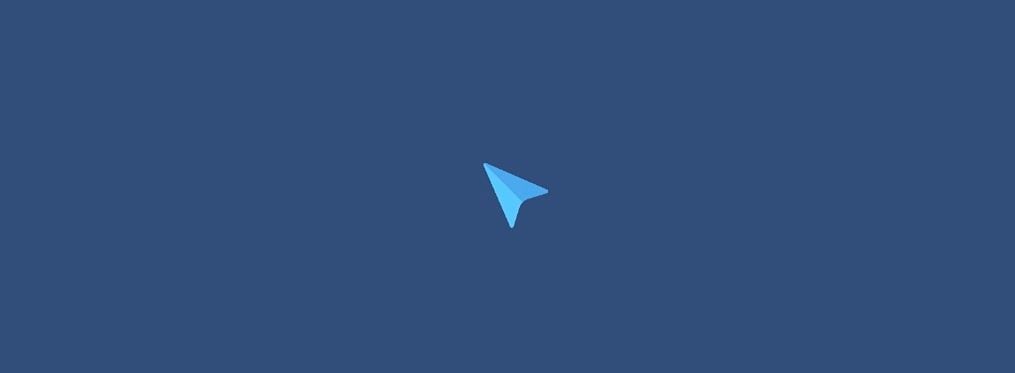
The Locked Cursor Lock Mode will force the cursor into the centre of the screen and hide the hardware cursor. You’ll have to take my word for it that this sprite cursor, that’s following the mouse, can’t be moved.
Typically you might lock the cursor whenever you want to control an object in your game, such as a camera or the player’s head, but without using a cursor on the screen to do so.
This works because the mouse’s delta movements, the directional movements of the mouse, are still recorded and can be used in the game, even though the cursor itself is locked.
Like this:
public Vector2 mouseDelta;
void Start()
{
UnityEngine.Cursor.lockState = CursorLockMode.Locked;
}
void Update()
{
mouseDelta = new Vector2(Input.GetAxis("Mouse X"), Input.GetAxis("Mouse Y"));
}
Or, if you’re using Unity’s new Input System,
Like this:
mouseDelta = Mouse.current.delta.ReadValue();
Which allows you to use the mouse for movement, even though the mouse cursor itself can’t move.
When limiting the movement of the mouse like this, since it can’t be used as a pointer, locking the cursor will also automatically hide it.
This happens regardless of whether or not the cursor is set to be visible using the cursor visible property, meaning that, even if you try to force the cursor to appear, it will always remain hidden when locked.
How to stop the mouse from leaving the screen
Setting the Cursor Lock State to Confined will limit the mouse’s movement so that it can’t move beyond the edges of the screen.
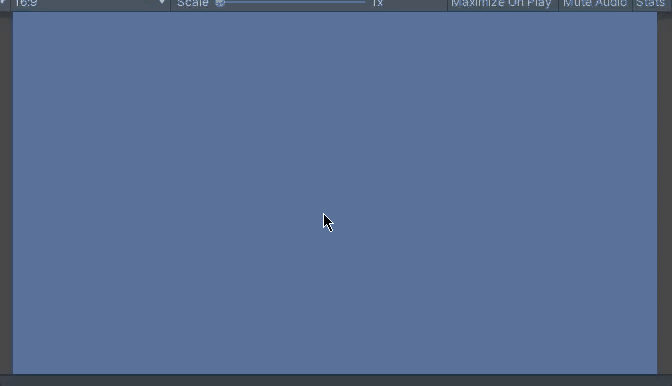
The Confined Cursor Lock Mode prevents the mouse from moving past the edges of the screen, but only on Windows or Linux.
You’ll still have full movement of the mouse, and you’ll be able to see the cursor, the only difference is that you won’t be able to move it outside of the game’s window.
Keep in mind, however, that the confined lock mode only works on Windows and on Linux.
Meaning that, if you’re building your game on a mac, it won’t appear to work.
Changing the Cursor Lock Mode when the application is focussed
Both Unity and the operating system are able to change the lock state of the cursor.
For this reason, Unity recommends that you check and set the lock state whenever the application regains focus.
This can be done using the On Application Focus event message, which will pass a boolean parameter that specifies if the application has gained focus or has lost it.
Like this:
public class LockCursor : MonoBehaviour
{
void OnApplicationFocus(bool hasFocus)
{
if (hasFocus)
{
Cursor.lockState = CursorLockMode.Locked;
Debug.Log("Application is focussed");
}
else
{
Debug.Log("Application lost focus");
}
}
}
When changing the lock state of the cursor, it can sometimes be tricky to know for sure if it’s working in the way you expect it to.
This is because, when in the editor, unlike in a built project running in the standalone player, focusing the application works in a slightly different way.
For example, pressing Escape in the Unity Editor will cause the game view to lose focus, releasing the cursor if it’s locked or hidden.
Clicking on the game view again will return focus to it, locking the cursor or hiding it.
This behaviour is normal and is, in fact, required.
After all, when you’re restricting the movement of the cursor in play mode, you’re going to need a way to actually move the mouse to the play button once you’re done, allowing you to quit again.
How to fix the error ‘Cursor does not contain a definition for lockstate’
The “Cursor does not contain a definition for…” error is a surprisingly common issue that happens as a result of duplicating script names.
This is because, when you’re working with the cursor in Unity, either because you want to hide it or because you want to lock it in place, chances are that you may have made a script called “Cursor” to make your changes in.
Except that Unity already has a Cursor Class, which means that, by adding a second, you’re actually hiding the first, causing the error.
The easiest way to fix this is by simply renaming your script to something other than “Cursor” so that Unity can clearly tell the difference between the two classes.
However, to avoid script naming issues in the first place, you could place your own class inside a namespace.
Like this:
namespace MyScripts
{
public class Cursor : MonoBehaviour
{
// Script things...
}
}
Separating your scripts using namespaces avoids potential conflicts before they happen.
Then, when you want to use a script that’s inside your namespace, just add your using directive to the top of the script.
Like this:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using MyScripts;
This works, except that, when dealing with this particular problem, if you need to use the Unity Engine namespace, which contains the cursor class, and your own namespace, which may contain your own cursor script, at the same time, Unity still won’t know which one to use.
In which case you may need to use an inline reference to the namespace you want instead.
Like this:
UnityEngine.Cursor.lockState = lockMode;
Now it’s your turn
Now I want to hear from you.
Are you locking the cursor in your game?
Or are you hiding it?
And what type of game are you making that means you need to restrict the movement of the cursor in the first place?
Whatever it is, let me know by leaving a comment.
Comments
Please write a article on wheel collider.
Thanks you
Hello John.
I need your advice on how to properly make the cursor in my 2D turn based strategy. I have 2 options to choose from:
1. As part of the UI. It’s a simple implementation, but it’s tricky to handle hover afterward.
2. Do as GameObject with collider and handle colissions by tags. But, for example, there will be a problem when the camera zooms in – the GameObject of the cursor will also zoom in.
I can’t decide how to do it right. So far, everything is moving towards the fact that both methods will have to be combined, i.e. process a GameObject without a sprite attached to the cursor, and then pass the UI data and change the sprite there.
What do you recommend?)
It depends a lot on how you need it to work but the solution I would probably choose is to create a cursor in the UI or use the hardware cursor with a replacement texture and then I’d handle interaction with the world using raycasts. That way, you won’t need to make anything work in a way it’s not supposed to. Let me know if that would work or if I’ve misunderstood.
I overlooked that i can customize the standard system cursor. I chose this path.) Next, I will use your advice from the link above.) Thank you so much!
You’re welcome!
Okay, now lets see you hide the cursor when hovering over a control in an EditorWindow [I’m using Handles.WireDisc to render a circle on the EditorWindow which works fine]. I have checked to see if the mouse position is within the bounds of the rectangle that defines my canvas on the EditorWindow – this works too, however Cursor.visible = false does not hide the cursor.
Just wanted to create a “CursorService”, a collection of features, to switch cursor … but Cursor.visible = true, just does not work…
Now that must be the reason, focus setting….
But then how do we make a fixed crosshair? Just a big image with a crosshair on it? (I will google it, but this cursor topic is just not as straightforward I would think.)
To make a fixed crosshair, you may want to consider locking and hiding the cursor and then using a UI image in the center of the screen to create the crosshair
Thank you.
This was very helpful, thank you so much!
really wish the syntax was easier, but hey