Virtual and Override are access modifiers that are used in Inheritance to allow classes to extend or modify derived functions.
For example, a base class and an inheriting class might both use some kind of Awake function, which can be a problem as one function of the same type will normally hide the other, stopping it from being called.
The Abstract, Virtual, Override and Sealed modifiers can be used to manage the relationship between hierarchical classes.
These modifiers are used in addition to access modifiers, so you’ll commonly see them with public, private and protected keywords, not instead of them, and they’re typically used to describe a function’s relationship with the class that it inherits from or with classes that may inherit from it.
How virtual and override work
To override a function from a base class, the function that’s going to be overridden must be Virtual.
This is what allows it to be replaced by a function in another class.
Like this:
public class Shape : MonoBehaviour
{
public virtual void CountSides()
{
Debug.Log("I don't know how many sides I have!");
}
}
It also needs to be either Public or Protected, so that deriving classes can access it.
Next, the derived function that’s going to replace the original must use the Override keyword.
Like this:
public class Square : Shape
{
public override void CountSides()
{
Debug.Log("I have 4 sides!");
}
}
If it doesn’t, then it won’t be used in place of the virtual method and the base version of the function will be called instead.
Then, even if you only have a reference to a type of shape, the specific type’s override function will be called in place of the original.
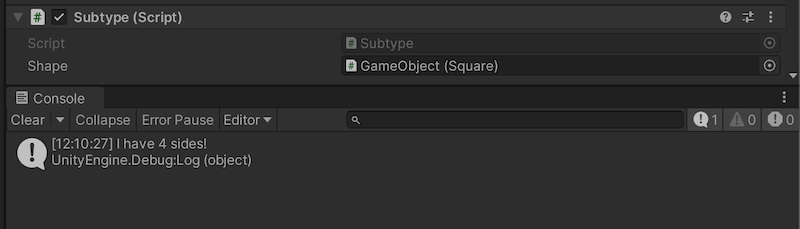
Because the Square class uses an overriding version of the Shape’s virtual function, that’s the version of the method that’s called.
It’s important to remember that overriding doesn’t extend the base function, it completely replaces it, meaning that the original will not be called.
Which can sometimes be useful.
But, if you do still want to call the base version of the function, along with the override replacement, then you can. You just have to do it manually.