Get Component is a method for finding a specific type of component on an object in Unity. The function searches for a component of a specific type and returns it if it finds one. It’s typically used for creating references between scripts and components when they are first created or during the game when they interact. While it is a necessary function, it can sometimes be slow
So how does Get Component work in Unity?
How Get Component works
Get Component takes a Generic Type which is the type of component or script that Get Component will look for on the object.
GetComponent<T>();
Meaning that, to find a particular type of component, you’ll need to replace T with whatever component type (or class name) that you’re trying to find.
Like this:
GetComponent<AudioSource>();
A common use of Get Component is to set up a reference variable automatically when a script is first loaded, such as in the Awake function.
Like this:
public class PlayerHealth : MonoBehaviour
{
AudioSource playerAudioSource;
void Awake()
{
playerAudioSource = GetComponent<AudioSource>();
}
}
Alternatively, it’s possible to use Get Component on different objects to check for specific functionality.
How to use Get Component on a different object
When no object reference is used, Get Component is called on the object that the script it’s called from is attached to.
However, it’s also possible to call Get Component from a different object using its reference or from an object that a component is attached to, by using a component or script reference.
Like this:
audioSource.GetComponent<PlayerHealth>(); // Gets a player health script on the same object as the audio source
otherObject.GetComponent<AudioSource>(); // Searches for an audio source on the Other Game Object
The return value of the Get Component function can be used to set a reference variable.
Like this:
public AudioSource playerAudioSource;
void Awake()
{
playerAudioSource = otherGameObject.GetComponent<AudioSource>();
}
However, this only works if the object actually has a component of that type.
If it doesn’t, the Get Component function will return null, meaning that if you try to access the set variable, you’ll get an error.
To avoid this, it’s usually a good idea to check and see if the reference you tried to set is null or not before trying to use it.
Like this:
public class Enemy : MonoBehaviour
{
[SerializeField] float enemyDamage = 20;
private void OnTriggerEnter(Collider other)
{
if (other.tag == "Player")
{
PlayerHealth playerHealth = other.GetComponent<PlayerHealth>();
if (playerHealth != null)
{
playerHealth.PlayerHurt(enemyDamage);
}
}
}
}
In more recent versions of Unity, it’s possible to try to get the component first and then only do something with it if it exists, all in a single function by using Try Get Component.
Like this:
public class Enemy : MonoBehaviour
{
[SerializeField] float enemyDamage = 20;
private void OnTriggerEnter(Collider other)
{
if (other.tag == "Player")
{
if (other.TryGetComponent(out PlayerHealth playerHealth))
{
playerHealth.PlayerHurt(enemyDamage);
}
}
}
}
In this example Try Get Component will search the object and, if a Player Health component exists, it will return true and pass the component it finds out to the local variable that’s specified when calling method.
This variable then can be used in the body of the if statement, but only if it actually exists.
If it doesn’t, Try Get Component returns false, and the body of the if condition is never executed, avoiding an error.
How to access components in child objects and parent objects
By default, Get Component only searches the object that it’s called on.
But, sometimes, depending on how your objects are structured, you might want to get a reference to something on a related object, such as one of its children or its parent using Get Component in Children or Get Component in Parent.
So how do these functions work?
How to use Get Component in Children
Get Component in Children works in the same way as Get Component except, instead of only checking the specified game object, it checks any child objects as well.
Like this:
public class PlayerHealth : MonoBehaviour
{
AudioSource playerAudioSource;
void Start()
{
playerAudioSource = GetComponentInChildren<AudioSource>();
}
}
Note that Get Component in Children checks its own game object and all of its children.
This means that if there’s already a matching component on the game object that calls it, this will be returned and not the component on the child object.
How to use Get Component in Parent
Just like Get Component in Children, you can also get components from parent objects as well.
Like this:
public class PlayerHealth : MonoBehaviour
{
AudioSource playerAudioSource;
void Awake()
{
playerAudioSource = GetComponentInParent<AudioSource>();
}
}
Alternatively, if you want to get a component from just the Root Object, and you don’t need to check any other objects along the way, it can sometimes be easier to simply reference that object directly.
This can be done using a transform shortcut that will return the topmost Transform Component in the hierarchy.
Like this:
playerAudioSource = transform.root.GetComponent<AudioSource>();
Just like Get Component in Children, Get Component in Parent checks both the game object it’s called on and the object’s parent.
So if there’s already a matching component on the game object, it will be returned just as if you were using the standard Get Component method.
This can be an issue when you are using multiple components of the same type and you want to be able to distinguish between them.
So how can you get just one component from an object?
How to choose which component is returned when using Get Component
Get Component, Get Component in Children and Get Component in Parent are great for retrieving a single component from another game object.
However…
If you’re using multiple components of the same type on an object, it can be tricky to know which component is actually going to be returned.
By default, when using any of the Get Component methods, Unity will return the first component of that type, from the top down, starting with the game object it’s called on.
That means that if there are two components of the same type, the first one will be returned.
But what if you want to access the second component?
Or the third?
A simple fix is to simply reorder the components so that the one that you want is first.
But, chances are, if you’ve got multiple components of the same type on a game object, you probably need to work with all of them.
For example, stitching audio clips together requires two audio sources to work properly, meaning that you need to have a reference to both of them and toggle between them.
On solution is to use Get Components to retrieve all of the components of a given type from a game object and then choose the one you want.
So how does that work?
First, create an array of the type of component you want to get a reference to:
Like this:
[SerializeField] AudioSource[] audioSources;
The square brackets after the variable type mark this variable as an Array.
Next, populate the array using GetComponents, GetComponentsInChildren or GetComponentsInParent.
Like this:
audioSources = GetComponents<AudioSource>();
This will add a reference to the array for every matching component that’s found.
Once you’ve filled the array, each element has its own index, starting from Zero:
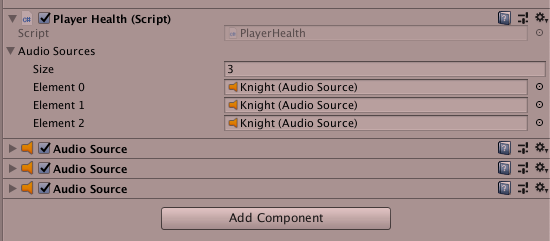
Each Array element has an index, starting from Zero, which is the first.
To access a particular element of the array, simply type the name of the array variable, followed by the index of the element you want to access in square brackets.
Like this:
public class PlayerHealth : MonoBehaviour
{
public AudioSource[] audioSources;
public AudioSource playerAudioSource;
void Start()
{
audioSources = GetComponents<AudioSource>();
playerAudioSource = audioSources[1];
}
}
Is Get Component Slow?
While Get Component isn’t inherently slow, it’s an operation that can, potentially, take a long time, depending on the number of objects and components that it needs to search through, and how frequently it’s called.
For this reason, it’s generally good practice to only use Get Component for one-off operations or to cache new references which can then be used repeatedly.
Like this:
public class Movement : MonoBehaviour
{
Rigidbody rb;
void Awake()
{
rb = GetComponent<Rigidbody>();
}
void FixedUpdate()
{
rb.AddForce(transform.up * 10);
}
}
However, so long as you’re not calling Get Component constantly, or calling it from a large number of objects all at once, you’re unlikely to run into performance issues when using it.
See also: