A For Loop in Unity is an iteration statement that allows you to execute the same block of code multiple times.
The for loop statement is made up of three elements:
- The Initialiser
- The Condition
- The Iterator
The Initialiser is called at the start of the loop and is typically used to declare the variable that will act as the loop’s dynamic data, such as an integer that’s used to identify a particular element of an array.
The Condition is the statement that will need to be true in order for the loop to continue. It is evaluated before each execution of the loop.
The Iterator is called after each iteration and is typically used to increment the dynamic value.
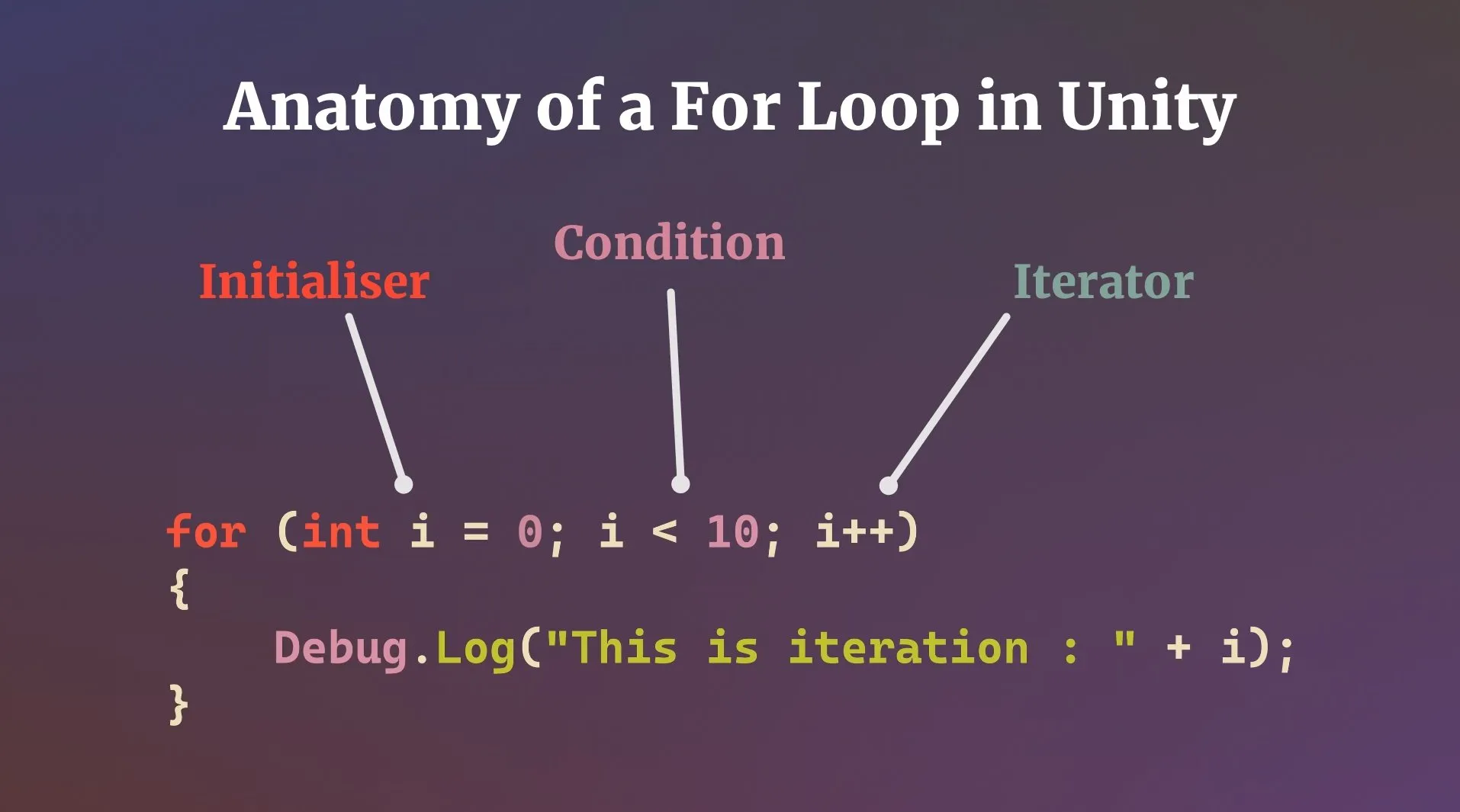
The For Loop statement is made up of three optional parts: the Initialiser, the loop Condition, and the Iterator.
Common uses of for loops include applying logic to multiple elements in an array or list, or performing an action a set number of times.
In both cases, it’s important to use the dynamic value, the iterator, in the loop’s condition. This is because it is possible for a for loop to process infinitely, causing the game to freeze.
This happens because the for loop will typically be processed on a single thread, meaning that it’s not possible for other logic to affect the condition of the loop. As a result, the action of the loop must affect the condition that allows it to finish executing.
for(int i =0; i < 10; i++)
{
// Do something 10 times
Debug.Log("This is iteration : " + i);
}