Making a light flicker in Unity can be a pretty simple task.
In fact, it can be as easy as turning a Light Component on or off as needed, disabling the light and creating a blinking effect.
But, there are lots of different reasons you might want to make a light flash in Unity.
You might be trying to create a blinking light, that turns on and off at steady intervals.
Or, you might want a light that flickers randomly, such as for an electrical effect, or for a faulty bulb.
Maybe you don’t want to turn the light on and off at all, maybe you want to change it smoothly over time, to create the kind of light that comes from a gentle flickering flame.
So, while making a light flash can be very easy, getting the exact effect that you want can be a little more tricky.
In this article, you’ll learn the different methods for making a light that flickers in Unity, so that you can pick the one that’s right for your project.
However, to make a light flicker at all, you’re going to need to turn it off, so what’s the easiest way to do that?
How to make a light blink on and off in Unity
The basic method of making a light blink or flash in Unity is very straightforward.
Just disable the Light Component to turn it off, and enable it again to turn it on.
Like this:
Light myLight;
void Start()
{
// Turns the light on
myLight.enabled = true;
// Turns the light off
myLight.enabled = false;
}
Simple, right?
Then, to make the light blink at a set interval, simply measure the amount of time that has passed each frame using a simple timer.
Once the timer’s duration is longer than the interval you want, you can toggle the light by setting it to the opposite of its current state.
Like this:
public Light myLight;
public float interval = 1;
float timer;
void Update()
{
timer += Time.deltaTime;
if (timer > interval)
{
myLight.enabled = !myLight.enabled;
timer -= interval;
}
}
In this example, the timer is reset by subtracting the interval amount from the time that’s elapsed.
This is generally more accurate than setting the timer to zero, as it accounts for the amount of time that has passed during the frame when the timer is reset.
Which results in a nice, steady blink.
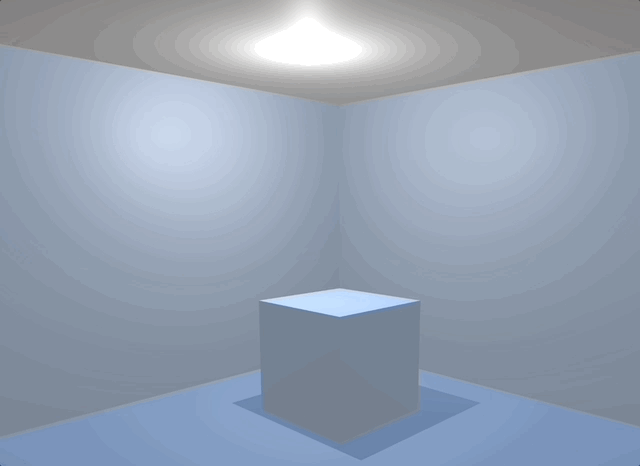
A basic blinking light effect typically involves toggling the light component on and off.
But what if you don’t want a steady blink?
What if you want to flicker a light randomly?
How to make a light flicker randomly
Making a light flicker at random intervals in Unity involves pretty much the same process as creating a steady blinking light.
The only thing that’s different is that the time interval is random instead of constant.
Like this:
public Light myLight;
float interval = 1;
float timer;
void Update()
{
timer += Time.deltaTime;
if (timer > interval)
{
myLight.enabled = !myLight.enabled;
interval = Random.Range(0f, 1f);
timer = 0;
}
}
This will turn the light on and off at random intervals within a set range.
Which looks like this:
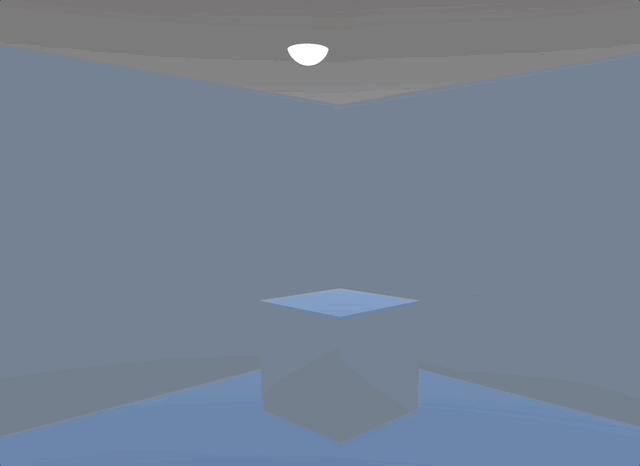
Blinking the light on and off randomly creates a kind of flickering effect.
However, while this does create a randomised blinking effect, real lights don’t always flicker in this way.
Typically, when a light flickers, it’s either on and then briefly turns off, or it’s off and then it briefly turns on.
But, in both cases, the duration of the actual flicker is a usually less than the interval between them.
Meaning that, if you want to create a more convincing flickering effect, you’ll need to keep track of two different time values, one for the amount of time a light can blink for, the Flicker Time, and one for the delay between flickers, the Wait Time.
Like this:
public Light myLight;
public float maxWait = 1;
public float maxFlicker = 0.2f;
float timer;
float interval;
void Update()
{
timer += Time.deltaTime;
if (timer > interval)
{
ToggleLight();
}
}
void ToggleLight()
{
myLight.enabled = !myLight.enabled;
if (myLight.enabled)
{
interval = Random.Range(0, maxWait);
}
else
{
interval = Random.Range(0, maxFlicker);
}
timer = 0;
}
Whether the light is on and flickers off, or is off and flickers on, depends on where, in the script, you toggle the light itself.
If you toggle the light before checking its status, as in the example above, the light will stay on, occasionally switching off.
Toggle it after, and the light will stay off, briefly blinking on now and then.
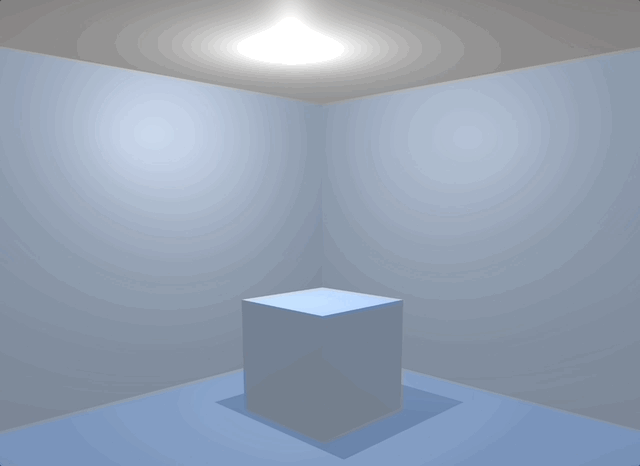
Turning off the light for a shorter amount of time than the interval between blinks can create a more realistic flickering effect, if that’s the kind of effect you want to create.
This effect is already a little more realistic than blinking the light at random intervals, but when real lights flicker the effect is usually more subtle than this.
So how can you make a more realistic flicker effect?
How to make a realistic flickering light effect in Unity
Sometimes, a light that’s malfunctioning will flicker by turning completely on or off.
And if that’s the effect that you want, then there’s nothing wrong with it.
However, when a real light is faulty, the flickering effect might be much more subtle than that.
For example, a real flickering light might not turn off completely, it might only dim.
Or, even if it does, it might flicker so fast, that it never appears to be fully off.
And, when it does flicker it might do it in short bursts, instead of one-off flashes.
Like this:
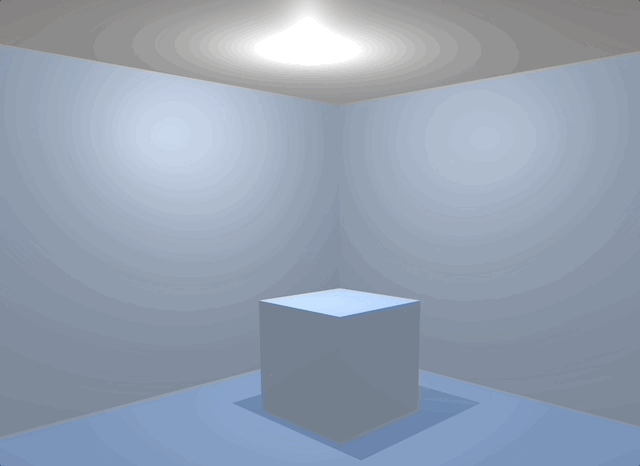
Dimming the light and flickering the light in bursts can make the effect more realistic.
So how can you change your flickering light to behave like that?
How to create a subtle flickering effect
To create a light that doesn’t turn off completely when it flickers, you’ll need to modify its intensity, instead of disabling the light component.
To do this, you’ll need to store the original intensity value of the light, so that you can turn the light back on again, and keep track of when it is considered to be ‘off’ by using a boolean value.
Then, when the light is off, simply switch the intensity to a random value that’s lower than the light’s normal brightness.
Like this:
public Light myLight;
public float maxInterval = 1;
public float maxFlicker = 0.2f;
float defaultIntensity;
bool isOn;
float timer;
float delay;
private void Start()
{
defaultIntensity = myLight.intensity;
}
void Update()
{
timer += Time.deltaTime;
if (timer > delay)
{
ToggleLight();
}
}
void ToggleLight()
{
isOn = !isOn;
if (isOn)
{
myLight.intensity = defaultIntensity;
delay = Random.Range(0, maxInterval);
}
else
{
myLight.intensity = Random.Range(0.6f, defaultIntensity);
delay = Random.Range(0, maxFlicker);
}
timer = 0;
}
This creates a more subtle effect than disabling the light completely, especially if the duration of the flicker is very short, as it gives the impression that the light is flashing faster than the framerate of the game.
How to make a soft flickering flame light
It’s possible to change the intensity of a light over time, so that it flickers slowly.
Meaning that, instead of a digital, electrical flicker, you get a smooth, dancing flame.
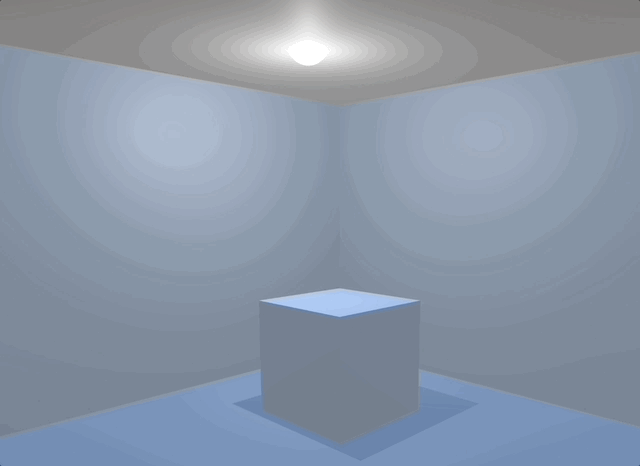
It’s possible to create a soft light flicker by changing the intensity of a light over time.
This works by randomly changing the target intensity of the light and then changing the actual brightness towards the target over time using Lerp.
Like this:
myLight.intensity = Mathf.Lerp(myLight.intensity, targetIntensity, timer / interval);
For extra realism, it’s also possible to move the light around as it flickers, causing real-time shadows close to the source to dance around in response to the moving flames.
Like this:
public Light myLight;
public float maxInterval = 1f;
float targetIntensity;
float lastIntensity;
float interval;
float timer;
public float maxDisplacement = 0.25f;
Vector3 targetPosition;
Vector3 lastPosition;
Vector3 origin;
private void Start()
{
origin = transform.position;
lastPosition = origin;
}
void Update()
{
timer += Time.deltaTime;
if (timer > interval)
{
lastIntensity = myLight.intensity;
targetIntensity = Random.Range(0.5f, 1f);
timer = 0;
interval = Random.Range(0, maxInterval);
targetPosition = origin + Random.insideUnitSphere * maxDisplacement;
lastPosition = myLight.transform.position;
}
myLight.intensity = Mathf.Lerp(lastIntensity, targetIntensity, timer / interval);
myLight.transform.position = Vector3.Lerp(lastPosition, targetPosition, timer / interval);
}
How to make a light flicker in bursts
Electrical flickers sometimes happen in bursts, where a light will flicker on and off quickly for a short period of time.
It’s possible to create a similar effect in Unity by using a coroutine.
This works in pretty much the same way as the random flickering method used above, except that, instead of running in Update, the random flicker code is executed inside of a While Loop in a coroutine instead.
Like this:
public Light myLight;
public float maxInterval = 2;
public float maxBurst = 1f;
public float maxFlicker = 0.2f;
float defaultIntensity;
bool isFlickering;
float timer;
float interval;
private void Start()
{
defaultIntensity = myLight.intensity;
interval = Random.Range(0,maxInterval);
}
void Update()
{
if (!isFlickering)
{
timer += Time.deltaTime;
}
if (timer > interval)
{
interval = Random.Range(0, maxInterval);
timer = 0;
StartCoroutine(FlickerLight(Random.Range(0, maxBurst)));
}
}
IEnumerator FlickerLight(float duration)
{
isFlickering = true;
float totalTime = 0;
float flickerTimer = 0;
float flickerInterval = Random.Range(0, maxFlicker);
while (totalTime < duration)
{
totalTime += Time.deltaTime;
flickerTimer += Time.deltaTime;
if (flickerTimer > flickerInterval)
{
myLight.intensity = Random.Range(0.5f, defaultIntensity);
flickerInterval = Random.Range(0, maxFlicker);
flickerTimer = 0;
}
yield return null;
}
myLight.intensity = defaultIntensity;
isFlickering = false;
}
Each burst of flickers stops the timer, meaning that a set of flickers must finish before the timed interval to the next burst is started, preventing them from overlapping each other.
How to make a material’s colour flicker with a light
If the material of the in-game object that’s emitting light into your scene is Emissive or Unlit, as it may well be for a light object (to allow the light to remain bright in darkness) you may wish to change the colour of the light itself to match the light component when it flickers.
Generally, this works by getting a reference to the material instance through the object’s renderer.
Which, for an unlit shader, allows you to change the light’s colour directly.
Like this:
lightRenderer.material.color = Color.white * myLight.intensity;
However, to change a material’s emission colour, you’ll need to get the ID of the shader’s emission property first.
Like this:
int emissionPropertyID = Shader.PropertyToID("_EmissionColor");
You’ll then be able to change the colour of the emission using the Set Colour function.
Like this:
lightRenderer.material.SetColor(emissionPropertyID, Color.white * (myLight.intensity - 0.25f));
Because the intensity of the light and the intensity of the shader’s colour will typically appear to be different in the game, it can help to offset the colour with a modifier value, in this example 0.25.
This prevents the light material from looking darker than the actual light it’s emitting.
Now it’s your turn
Now I want to hear from you.
How are you modifying lights in your game?
Are you turning them on and off?
Or are you changing their intensity?
And what have you learned about working with lights in Unity that you know someone else would find useful?
Whatever it is, let me know by leaving a comment.
Comments
Thanks again John for another great down-to-earth post. Useful tips and self-contained examples, very helpful!
You’re welcome!
Thanks John!
Would you be able to do a tutorial on setting up lighting. For example creating a desk lamp or a street light or even like the ceiling light you have in the example above.
Thank you! I’ll see what I can do!